Harness Cloud Storage Power in Your Flutter Apps
Published on by Flutter News Hub
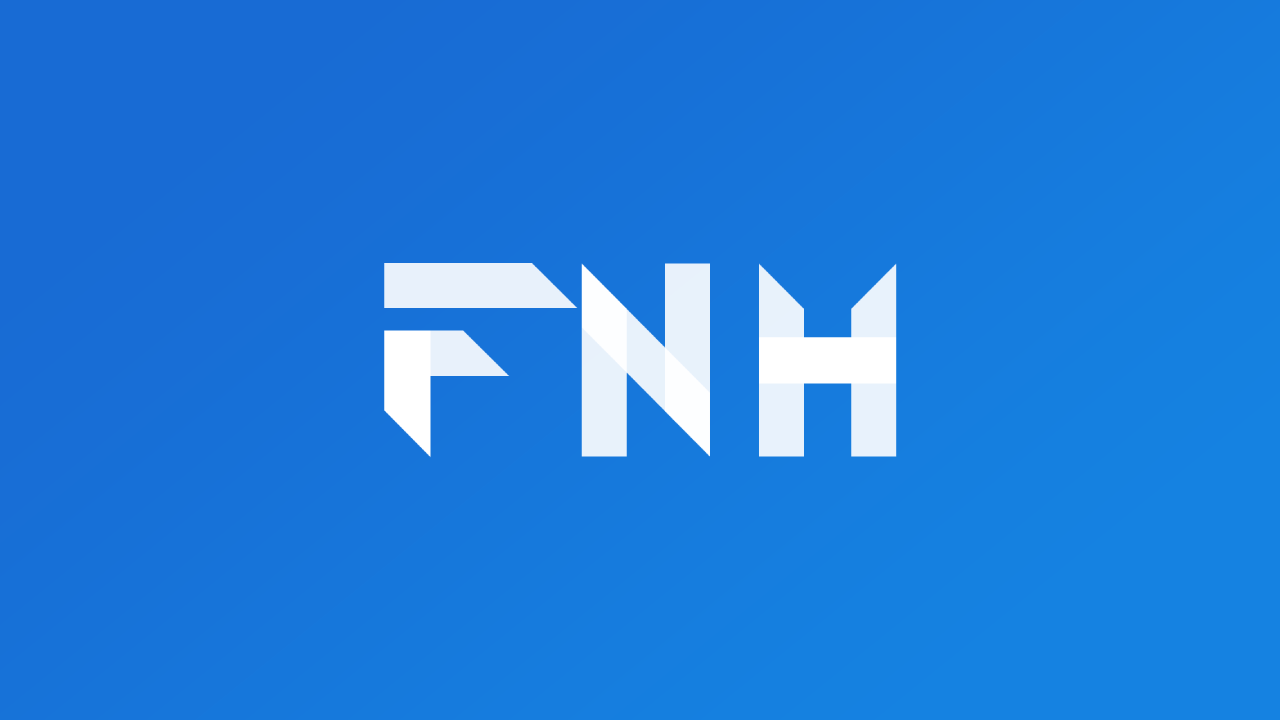
Firebase Cloud Storage for Flutter empowers you to effortlessly manage and store your app's data in the cloud. With this plugin, you can seamlessly upload, download, and manipulate files, ensuring secure and scalable storage for your app.
Getting Started
To kickstart your journey with Cloud Storage for Flutter, follow these simple steps:
import 'package:firebase_storage/firebase_storage.dart';
// Create a Firebase Storage instance
final storage = FirebaseStorage.instance;
// Create a reference to the file you want to upload
final storageRef = storage.ref().child('uploads/myImage.png');
// Upload the file
final uploadTask = storageRef.putFile(imageFile);
// Register for the upload's status changes
uploadTask.snapshotEvents.listen((snapshot) {});
File Management Made Easy
With Cloud Storage for Flutter, you can effortlessly manage your files:
// Get a reference to the file
final storageRef = storage.ref().child('uploads/myImage.png');
// Download the file
final downloadTask = storageRef.writeToFile(localFile);
// Register for the download task's status changes
downloadTask.snapshotEvents.listen((snapshot) {});
// Delete the file
storageRef.delete();
Metadata Management
Inspect and manipulate your files' metadata using Cloud Storage for Flutter:
// Get the metadata of the file
final metadata = await storageRef.getMetadata();
// Update the metadata of the file
storageRef.updateMetadata({
'customMetadata': {
'displayName': 'newDisplay'
}
});
Advanced Features for Real-Time Updates
Cloud Storage for Flutter offers real-time updates on file changes through events:
// Listen for file metadata changes
storageRef.metadataChanges().listen((metadata) {});
// Listen for file download progress
storageRef.downloadURL().then((url) {});
Next Steps
Dive into the complete documentation to unleash the full potential of Cloud Storage for Flutter:
Support and Contribution
For issues and feedback related to the plugin, visit the FlutterFire issue tracker.
For general Flutter issues, head to the Flutter issue tracker.
Feel free to contribute to the plugin by following the contribution guide.