GoRouter: A Comprehensive Guide for URL-Based Navigation in Flutter
Published on by Flutter News Hub
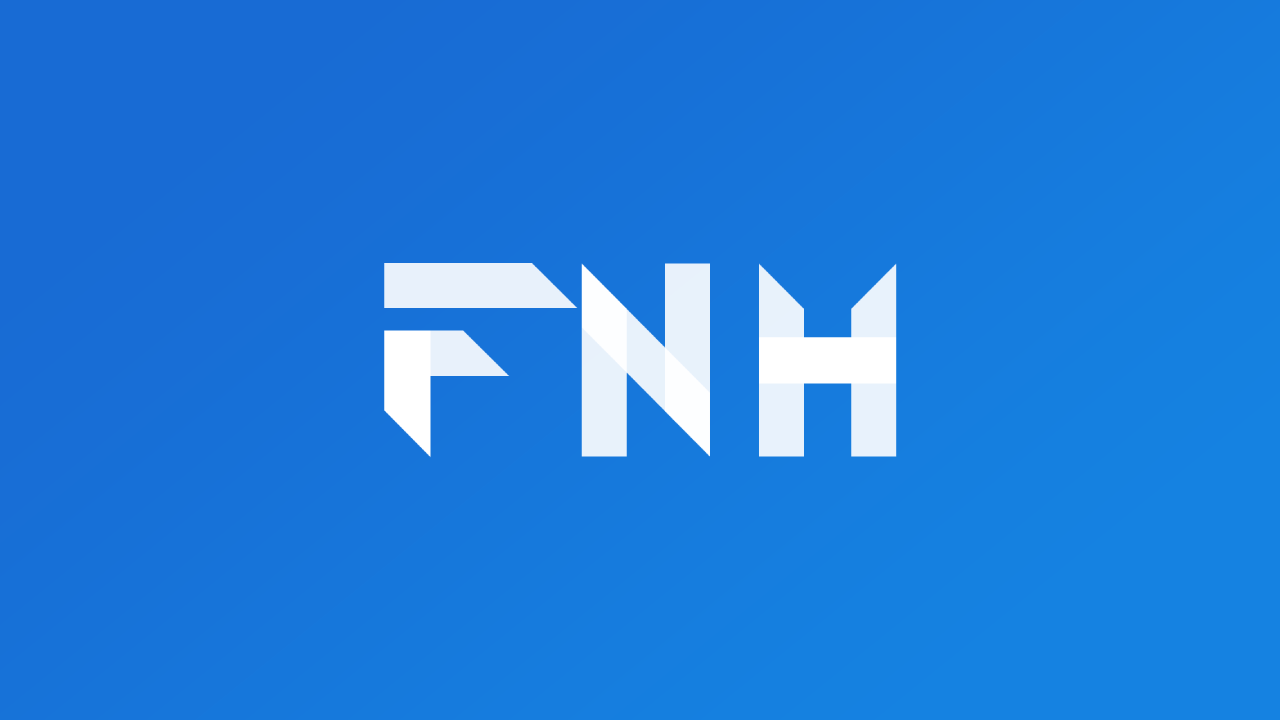
GoRouter is a declarative routing package for Flutter that enhances navigation with a convenient, URL-based API. It provides a robust feature set that simplifies screen navigation, parameter parsing, deep linking, and more.
Features of GoRouter
- URL-based Navigation: Define routes using URL patterns and navigate using a URL.
- Path and Query Parameter Parsing: Extract parameters from URLs using a template syntax, enabling dynamic content display.
- Sub-Routes: Display multiple screens within a single destination route.
- Redirection: Re-route users based on application state, such as authentication checks.
- ShellRoutes: Support for multiple Navigators, allowing for a persistent menu or bottom navigation bar.
- Material and Cupertino Compatibility: Works seamlessly with both Material and Cupertino design systems.
- Backwards Compatibility: Maintains compatibility with the Navigator API.
Getting Started
- Add GoRouter to your project's dependencies:
dependencies: go_router: ^latest_version
- Create a GoRouter instance:
final router = GoRouter( routes: [ GoRoute( path: '/home', builder: (context, state) => const HomeScreen(), ), ], );
- Use GoRouter in your app:
MaterialApp( home: GoRouterProvider( router: router, child: const MyHomePage(), ), );
Navigation
- Navigate to a route:
GoRouter.of(context).push('/home');
- Pop the current route:
GoRouter.of(context).pop();
- Replace the current route:
GoRouter.of(context).replace('/home');
Redirection
- Redirect users based on a condition:
final router = GoRouter( redirect: (context, state) { final isLoggedIn = context.read().isLoggedIn; if (!isLoggedIn) return '/login'; return null; }, routes: [ // ... ], );
Example: Implementing a Simple Navigation
// Main Screen class MainScreen extends StatelessWidget { const MainScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Main Screen'), ), body: Center( child: ElevatedButton( onPressed: () { GoRouter.of(context).push('/profile'); }, child: const Text('Go to Profile'), ), ), ); } } // Profile Screen class ProfileScreen extends StatelessWidget { const ProfileScreen({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Profile Screen'), ), ); } }
Conclusion
GoRouter simplifies navigation in Flutter, offering a powerful set of features for dynamic and user-friendly navigation experiences. Its URL-based API and comprehensive feature set make it an indispensable tool for any Flutter app developer.