Getting Started with sqflite: A Comprehensive Guide to SQLite on Flutter
Published on by Flutter News Hub
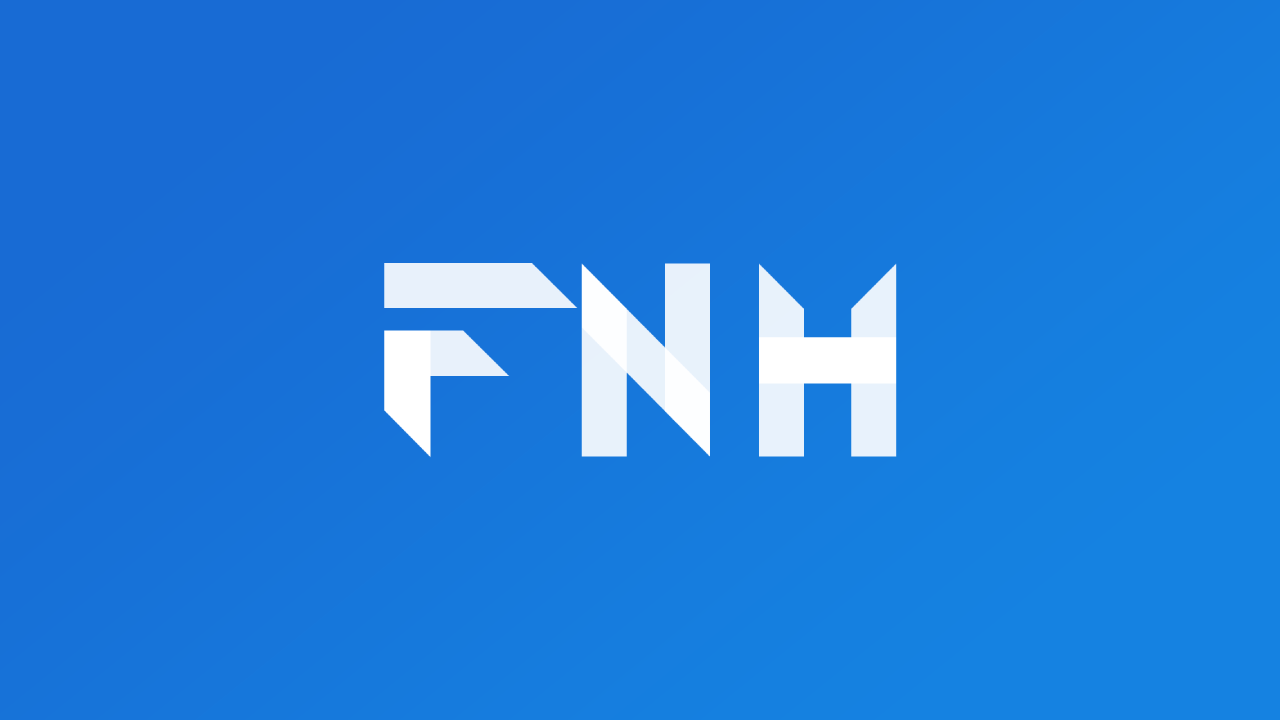
sqflite is a robust SQLite plugin for Flutter, providing seamless database management across iOS, Android, and macOS. This guide will walk you through the basics of using sqflite, from installation to managing tables and performing SQL queries.
Installation
To install sqflite, add the following line to your pubspec.yaml file:
dependencies: sqflite: ^latest_version
Run flutter pub get to install the package.
Opening a Database
Open a SQLite database by providing a path to the database file.
import 'package:sqflite/sqflite.dart'; var db = await openDatabase('my_database.db');
SQL Helpers
sqflite provides helper methods for CRUD (Create, Read, Update, Delete) operations, simplifying database interactions.
Inserting Data
await db.insert('table_name', { 'column1': value1, 'column2': value2, });
Querying Data
List results = await db.query('table_name');
Updating Data
await db.update('table_name', { 'column1': newValue1, }, where: 'column2 = ?', whereArgs: [oldValue2]);
Deleting Data
await db.delete('table_name', where: 'column1 = ?', whereArgs: [value1]);
Raw SQL Queries
For complex queries or direct SQL execution, use rawQuery.
List results = await db.rawQuery('SELECT * FROM table_name');
Transactions
Transactions provide atomic database operations, ensuring data integrity.
await db.transaction((txn) async { // Execute SQL within the transaction. });
Batch Support
Batches allow multiple operations to be executed efficiently.
var batch = db.batch(); batch.insert('table_name', {'column1': 'value1'}); await batch.commit();
Supported SQLite Types
sqflite supports the following SQLite data types:
- INTEGER (int)
- REAL (num)
- TEXT (String)
- BLOB (Uint8List)
Additional Features
- Encryption Support: Encrypt your database for enhanced security.
- Desktop Support: Use sqflite on desktop platforms like Linux, Windows, and DartVM.
- Web Support (Experimental): Access SQLite databases on the web.
Conclusion
sqflite empowers you to work with SQLite databases in Flutter with ease. Its comprehensive feature set, helper methods, and performance optimizations make it an indispensable tool for data management in your Flutter applications.