GetIt: A Comprehensive Overview for Efficient Service Management in Dart and Flutter
Published on by Flutter News Hub
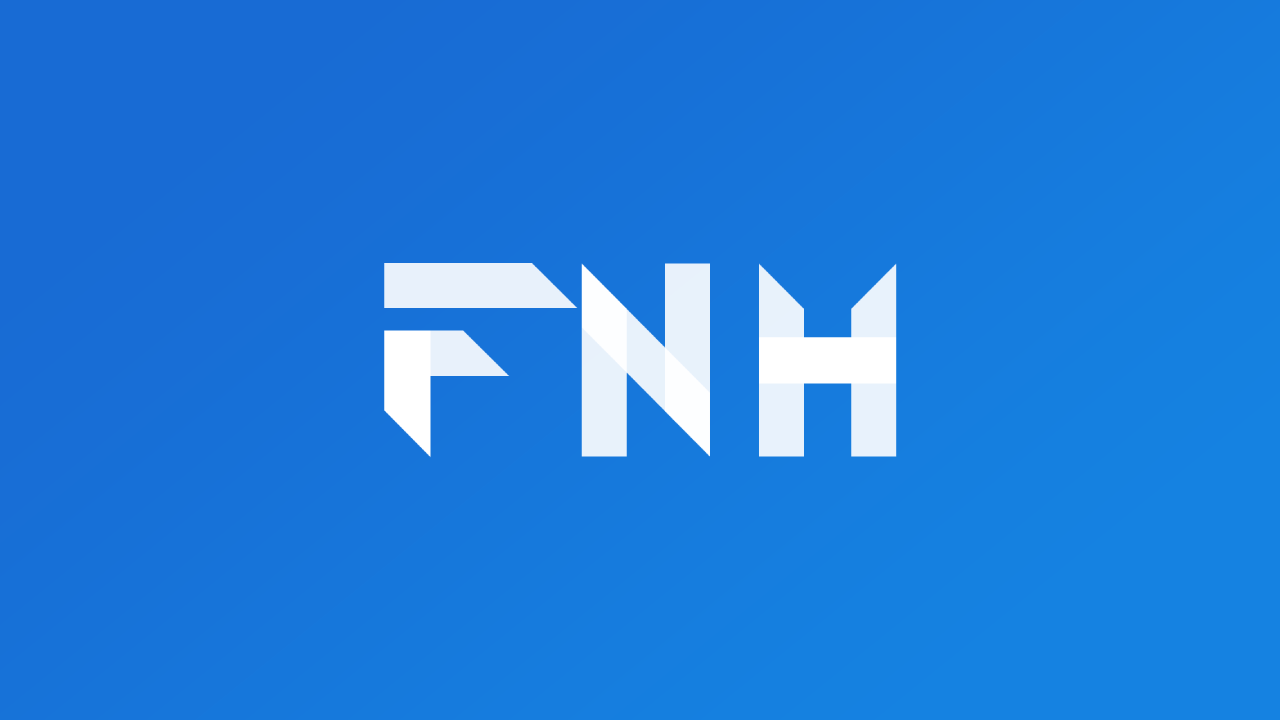
GetIt is a powerful Service Locator for Dart and Flutter applications that streamlines dependency injection and object access. This detailed guide will delve into its functionality, providing code examples and expert insights.
Key Features of GetIt
- Fast (O(1)) performance
- Easy to learn and use
- Facilitates object access from anywhere in an app
- Supports multiple ways of registration with varying lifetimes
- Automatic synchronization of asynchronous initializations
- Comprehensive testing capabilities
Getting Started with GetIt
To initialize GetIt, assign its instance to a global variable for convenient access.
final getIt = GetIt.instance;
Registering Objects:
- Singleton:
getIt.registerSingleton(AppModelImplementation());
- LazySingleton:
getIt.registerLazySingleton(() => RestAPIImplementation());
- Factory:
getIt.registerFactory(() => AppModelImplementation());
- Factory with Parameters:
getIt.registerFactoryParam((s, i) => UserParam(param1: s, param2: i));
- Singleton With Dependencies:
getIt.registerSingletonWithDependencies( () => AppModelImplmentation(), dependsOn: [ConfigService, DbService, RestService] );
Accessing Objects
To access registered objects:
- Singleton/LazySingleton/Factory without parameters:
var appModel = getIt();
- Factory with parameters:
var userParam = getIt(param1: 'abc', param2: 3);
- SingletonRegisteredByName:
var instance = getIt(instanceName: "restService1");
Asynchronous Initializations
GetIt supports asynchronous initializations of Singletons.
Asynchronous Singleton:
getIt.registerSingletonAsync(() async { final configService = ConfigService(); await configService.init(); return configService; });
Synchronizing Asynchronous Initializations:
- Using dependsOn:
getIt.registerSingletonAsync(createDbServiceAsync, dependsOn: [ConfigService]);
- Using isReady:
await getIt.isReady();
- Using allReady:
var allReady = getIt.allReady();
Advanced Features
- Named Registration: Register objects with unique names for each type.
- Accessing an Object by Runtime Type:
final instance1 = getIt.get(type: TestClass);
- Multiple Instances of GetIt:
GetIt myOwnInstance = GetIt.asNewInstance();
Conclusion
GetIt is an indispensable tool for managing dependencies and accessing objects in Dart and Flutter applications. Its ease of use, performance, and comprehensive features make it a valuable asset for any project. By leveraging its capabilities effectively, developers can create well-structured, loosely coupled codebases.