Flutter WeChat Assets Picker: A Comprehensive Guide
Published on by Flutter News Hub
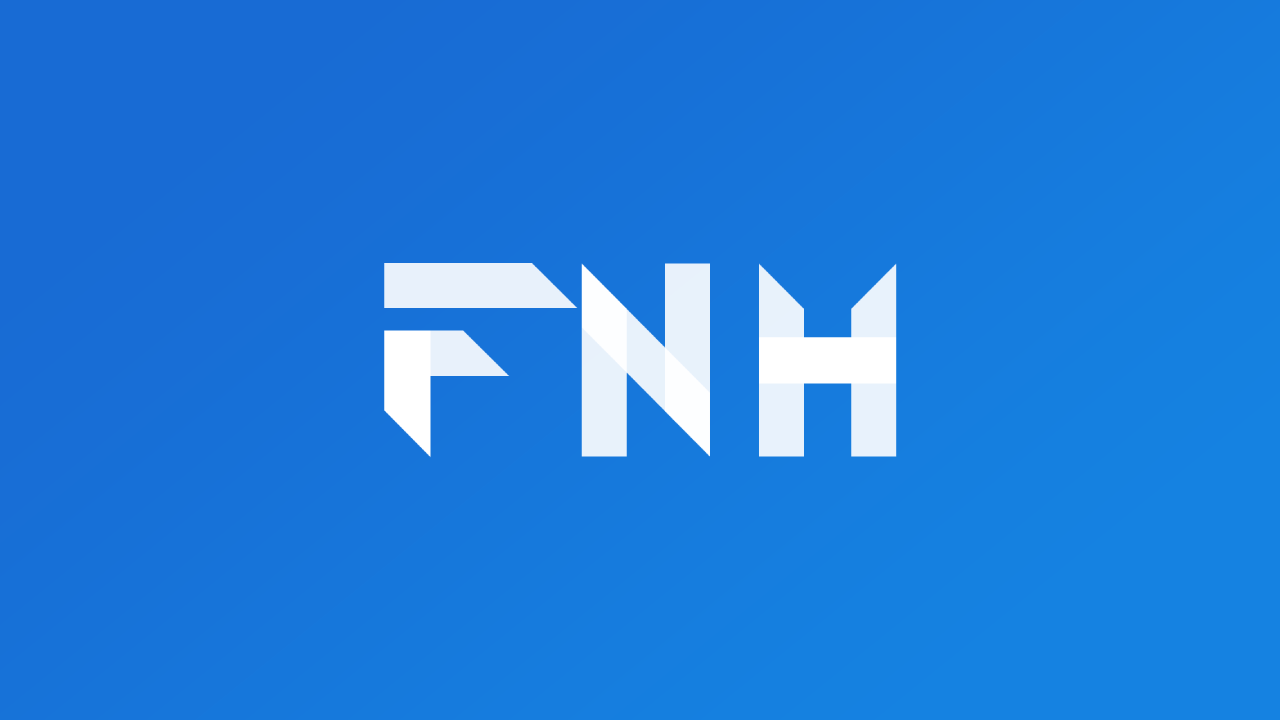
The WeChat Assets Picker for Flutter is a powerful library that allows developers to integrate WeChat-style image, video, and audio picking capabilities into their applications. With a highly customizable UI and a wide range of features, this library empowers developers to create intuitive and user-friendly media selection experiences.
Features
- Complete a11y support for TalkBack and VoiceOver
- Fully customizable with delegates override
- Fully customizable theme based on ThemeData
- Completely WeChat style (even more)
- Adjustable performance with different configurations
- Image support, including HEIF image type
- Video support
- Audio support (sandboxed on iOS and macOS)
- Single picking mode
- Internationalization (i18n) support
- RTL language support
- Special item builder support
- Custom sort path delegate support
- Custom text delegate support
- Custom filter options support
- macOS support
Installation
To use the WeChat Assets Picker, add the following dependency to your pubspec.yaml file:
dependencies: wechat_assets_picker: ^latest_version
Usage
To use the picker, simply call the AssetPicker.pickAssets method. You can pass in a variety of options to customize the picker's behavior, such as:
- selectedAssets: A list of initially selected assets.
- maxAssets: The maximum number of assets that can be selected.
- pageSize: The number of assets to load per page.
- gridThumbnailSize: The size of the thumbnails in the grid view.
- pathThumbnailSize: The size of the thumbnails in the path selector.
- previewThumbnailSize: The size of the preview thumbnails.
- requestType: The type of request to make (e.g., RequestType.image, RequestType.video).
- specialPickerType: A special picker type to be integrated (e.g., SpecialPickerType.noPreview).
- keepScrollOffset: Whether to keep the scroll offset between pushes and pops.
- sortPathDelegate: A delegate to sort paths by (e.g., CommonSortPathDelegate).
- sortPathsByModifiedDate: Whether to allow sort delegates to sort paths with FilterOptionGroup.containsPathModified.
- filterOptions: Custom filter options to apply to the picker.
- gridCount: The number of columns in the grid view.
- themeColor: The main theme color for the picker.
- pickerTheme: A custom theme to apply to the picker.
- textDelegate: A custom text delegate to use for the picker.
- specialItemPosition: A special position to place a special item in the picker.
- specialItemBuilder: A custom builder to use for the special item.
Examples
Here is an example of how to use the picker to select a single image:
final List? result = await AssetPicker.pickAssets( context, requestType: RequestType.image, maxAssets: 1, specialPickerType: SpecialPickerType.noPreview, );
Here is another example of how to use the picker to select multiple images and videos:
final List? result = await AssetPicker.pickAssets( context, maxAssets: 10, requestType: RequestType.common, );
Customization
The WeChat Assets Picker can be fully customized using a variety of methods. You can override the following delegates:
- AssetPickerBuilderDelegate
- AssetPickerViewerBuilderDelegate
- AssetPickerProvider
- AssetPickerViewerProvider
You can also use the pickerTheme parameter to apply a custom theme to the picker.
Tips
- When using the picker to select photos, videos, or audios, ensure that the correct permissions are declared in your AndroidManifest.xml file.
- On iOS, add the NSPhotoLibraryUsageDescription key to your Info.plist file to obtain the user's permission to access the photo library.
- To prevent duplicate selection, use the selectedAssets parameter to pass in the list of initially selected assets.
- Use the sortPathDelegate parameter to customize the order of the paths in the path selector.
- Use the filterOptions parameter to apply custom filter options to the picker.
- Use the specialItemBuilder parameter to add a custom special item to the picker.
Conclusion
The WeChat Assets Picker for Flutter is a powerful and versatile tool for selecting images, videos, and audios in your applications. With its highly customizable UI and wide range of features, this library enables developers to create user-friendly and efficient media selection experiences.