Flutter Slidable: A Comprehensive Guide for Mobile App Developers
Published on by Flutter News Hub
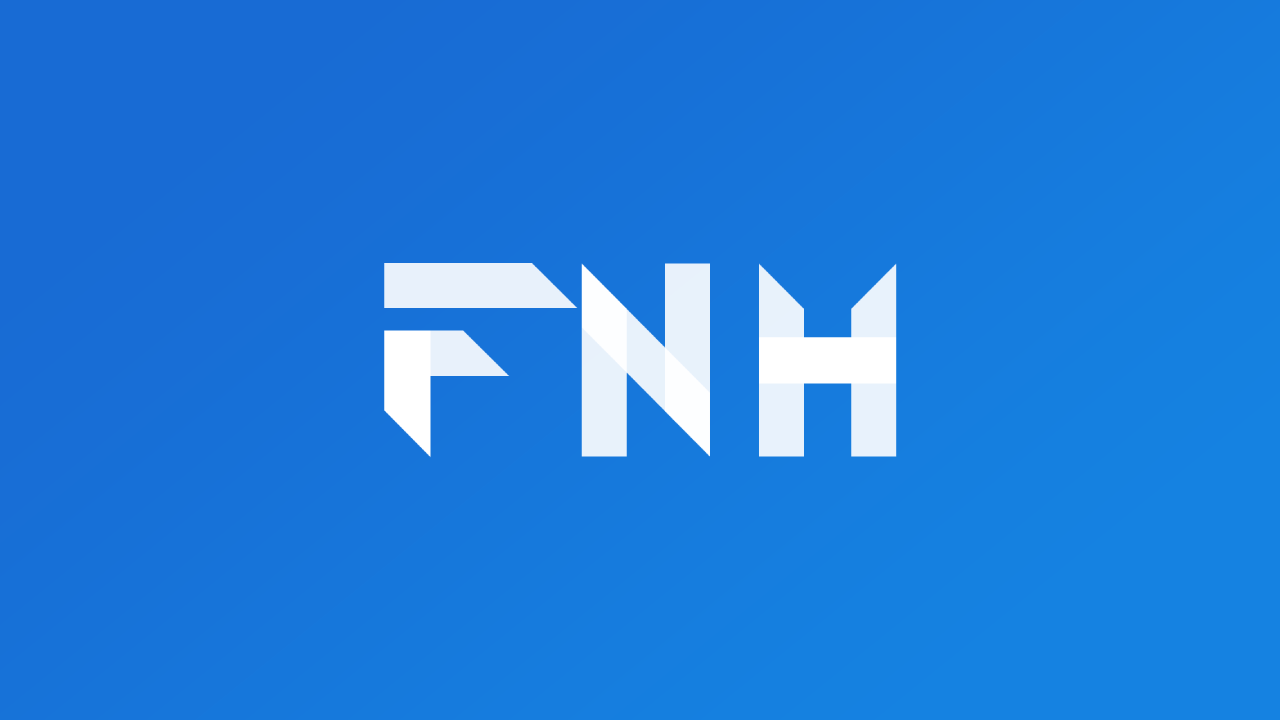
Flutter Slidable is a versatile Flutter package that empowers developers to create interactive and customizable list items that can be swiped to reveal actions. This guide will take you through the essential features, usage, and customization options of this powerful package.
Key Features
- Directional Slide Actions: Create actions that can be swiped from left/top or right/bottom.
- Dismissable Items: Allow users to dismiss items from the list by swiping.
- Built-in Action Panes: Use predefined action panes for common operations like delete, share, or archive.
- Customizable Animations: Design custom layouts and animations to suit your specific UI needs.
- Builder Support: Create dynamic slide actions with special effects during animation.
- Automatic Closing: Configure the Slidable to close when an action is tapped or when neighboring scrollable elements are interacted with.
Getting Started
To integrate Flutter Slidable into your project:
dependencies: flutter_slidable: ^latest_version
Import the package:
import 'package:flutter_slidable/flutter_slidable.dart';
Basic Usage
Create a Slidable widget to display a list item with slide actions:
Slidable( key: const ValueKey(0), // Key for dismissible items startActionPane: ActionPane( motion: const ScrollMotion(), // Animation for start actions children: [ SlidableAction( onPressed: () {}, // Action callback backgroundColor: Colors.red, foregroundColor: Colors.white, icon: Icons.delete, label: 'Delete', ), ], ), endActionPane: ActionPane( motion: const ScrollMotion(), // Animation for end actions children: [ SlidableAction( onPressed: () {}, backgroundColor: Colors.green, foregroundColor: Colors.white, icon: Icons.share, label: 'Share', ), ], ), child: const ListTile(title: Text('Slide me')), )
Motions
Flutter Slidable provides several built-in motions to control the animation of action panes:
- Behind Motion: Actions appear behind the Slidable.
- Drawer Motion: Actions slide out like drawers.
- Scroll Motion: Actions follow the Slidable's movement.
- Stretch Motion: Actions stretch as the Slidable moves.
Controller
Use the SlidableController to control the opening and closing of actions programmatically:
final controller = SlidableController(); // ... Slidable( controller: controller, // ... ); // ... void _handleOpen() { controller.openEndActionPane(); }
Customization
Flutter Slidable allows for extensive customization of its components:
Action Panes
- dismissible: Allow the pane to dismiss the Slidable.
- extentRatio: Set the width of the pane relative to the Slidable's size.
- motion: Specify the animation used for the pane's movement.
Slide Actions
- backgroundColor: Background color of the action.
- foregroundColor: Color of the action's icon and label.
- icon: Icon to display on the action.
- label: Text label to display alongside the icon.
- onTap: Callback when the action is tapped.
Conclusion
Flutter Slidable empowers you to create interactive and functional list items with slide actions. By leveraging its customizable features and built-in components, you can enhance the user experience of your Flutter applications with ease.