Flutter Pull to Refresh: A Comprehensive Guide
Published on by Flutter News Hub
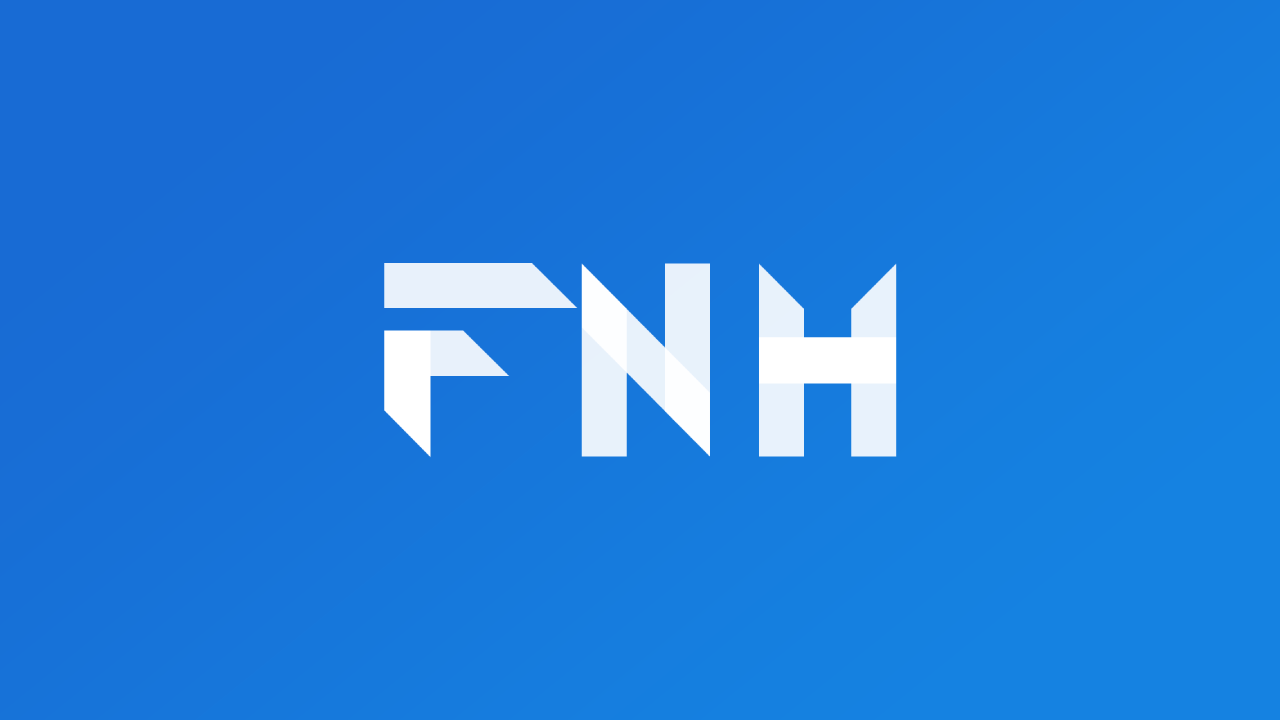
Flutter Pull to Refresh is a powerful widget that enables users to refresh and load more data in their Flutter applications. It supports both pull-down refresh and pull-up load functionality and can be integrated seamlessly into various scroll widgets.
Usage
To use Flutter Pull to Refresh, simply wrap your ScrollView widget (e.g., ListView, GridView) within the SmartRefresher widget.
import 'package:pull_to_refresh/pull_to_refresh.dart'; class MyHomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( body: SmartRefresher( controller: _refreshController, onRefresh: _onRefresh, onLoading: _onLoading, child: ListView.builder( itemCount: items.length, itemBuilder: (context, index) => Card(child: Text(items[index])), ), ), ); } }
Features
- Pull-down refresh and pull-up load: Easily implement both refresh and load more functionality.
- Compatible with various scroll widgets: Integrates seamlessly with ListView, GridView, CustomScrollView, and more.
- Customizable indicators: Choose from built-in indicators or create your own custom indicators.
- Global configuration: Set default properties and indicators for all SmartRefresher widgets in your app.
- OverScroll support: Control the overscroll distance and customize the springback animation.
- Horizontal and vertical refresh: Supports both horizontal and vertical scrolling for versatility.
- Two-level refresh: Implement multi-level refresh effects, similar to those found in WeChat and Taobao.
- Link indicator: Place the refresh indicator in a different location from the scroll view for a unique effect.
Custom Indicators
Flutter Pull to Refresh provides several built-in refresh indicators, such as ClassicIndicator and WaterDropHeader. You can also create your own custom indicators by extending the Indicator abstract class.
class MyCustomIndicator extends Indicator { @override Widget build(BuildContext context, IndicatorMode mode) { return Container( // Customize the indicator's appearance based on the mode (loading, refresh, etc.) ); } }
Global Configuration
To configure the default properties and indicators for all SmartRefresher widgets in your app, use the RefreshConfiguration widget.
class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return RefreshConfiguration( headerBuilder: () => WaterDropHeader(), footerBuilder: () => ClassicFooter(), child: MaterialApp( // Your app's routes and widgets ), ); } }
Performance Considerations
For optimal performance, it is important to avoid nesting SmartRefresher widgets within ScrollView widgets. This can cause scrolling issues and unexpected behavior. Instead, place SmartRefresher directly beneath the MaterialApp or CupertinoApp widget.
Additional Notes
- For a complete list of properties and usage examples, refer to the official Flutter Pull to Refresh documentation.
- If you encounter any issues, consult the FAQ and Troubleshooting guide.
- For more inspiration and customization options, explore the example applications provided in the GitHub repository.