Flutter and Material Design: Crafting Beautiful UIs
Published on by Flutter News Hub
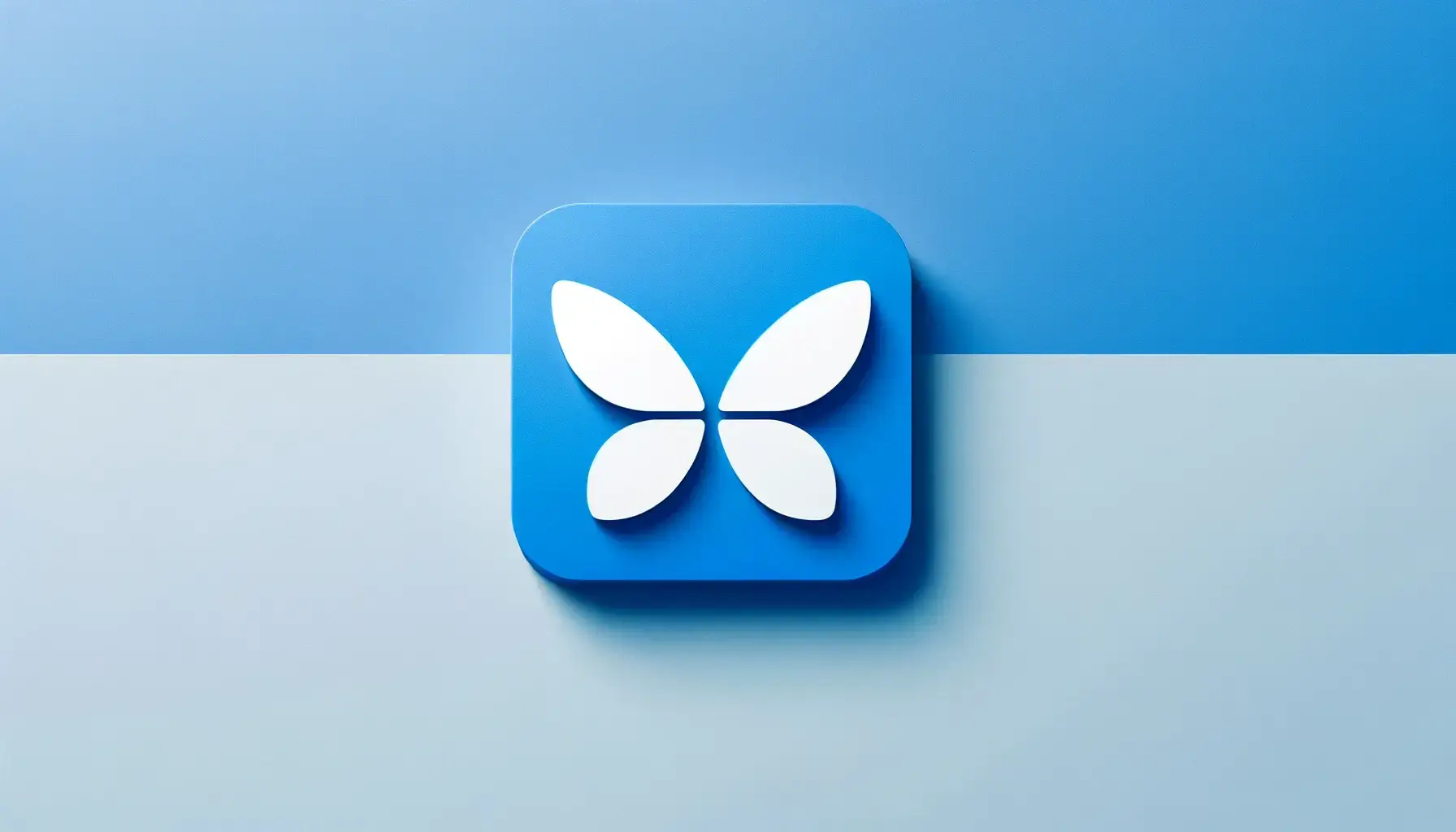
Flutter, Google's UI toolkit for crafting beautiful, natively compiled applications, is known for its fast development cycle, expressive and flexible UI, and native performance. One of the key features that make Flutter stand out is its seamless integration with Material Design, Google's design language. This blog post explores how Flutter and Material Design work together to create stunning user interfaces.
What is Material Design?
Material Design is a design language developed by Google in 2014. It's an innovative visual language that combines principles of good design with advancements in technology and science, creating a foundation for designing interfaces. Material Design focuses on providing a more intuitive and accessible user experience.
Flutter’s Material Design Widgets
Flutter comes packed with a rich set of pre-designed widgets that follow Material Design principles. These widgets are more than just UI components; they encapsulate all the Material Design specifications, such as layout, styling, and animation.
Some Key Material Widgets in Flutter:
- MaterialApp: The starting point of a Material Design app. It creates a number of useful widgets, including Navigator, which manages a stack of screens.
- Scaffold: Provides a basic Material Design layout structure, making it easy to implement drawers, snack bars, and bottom sheets.
- AppBar: A Material Design app bar that can be used to provide context about the current screen.
- FloatingActionButton: A circular icon button that hovers over content, commonly used for a primary action in the app.
- Drawer: A Material Design panel that slides in horizontally from the edge of a Scaffold to show navigation links in an application.
Implementing Material Design in Flutter Apps
When you create a new Flutter project, it automatically uses Material Design. Here's a simple example to illustrate how easy it is to create a Material Design interface:
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Material Design App'), ), body: Center( child: Text('Hello, Material Design!'), ), floatingActionButton: FloatingActionButton( onPressed: () {}, child: Icon(Icons.add), ), ); } }
Benefits of Using Material Design in Flutter
- Consistency Across Platforms: Material Design maintains visual consistency across different platforms and screen sizes.
- User Experience: Material Design's principles are based on real-world metaphors, making the UI intuitive and user-friendly.
- Customization: While Material Design provides a standard, it’s also flexible enough to allow for creative and brand-driven customization.
- Efficiency: With Material widgets, developers can create beautiful designs with less effort and time.
Conclusion
Flutter and Material Design are a powerful combination for developing modern, visually appealing applications. With Flutter's extensive range of Material widgets, developers can implement Material Design with minimal effort, ensuring an attractive and cohesive UI. Whether you're building for Android, iOS, web, or desktop, embracing Material Design in your Flutter apps can significantly enhance user experience and interface aesthetics.