Firebase Messaging Plugin for Flutter: Your Guide to Real-Time Communication
Published on by Flutter News Hub
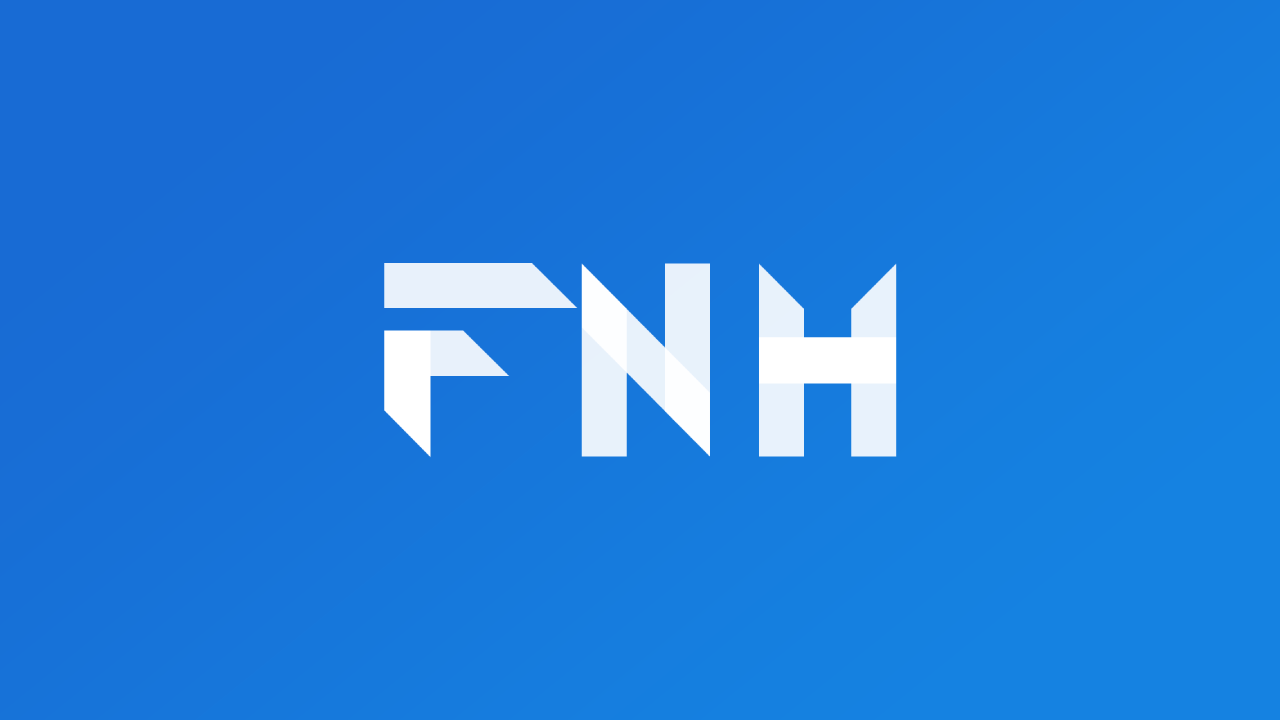
Unlock the Power of Real-Time Messaging for Your Flutter App
Firebase Cloud Messaging (FCM) is an indispensable tool for any modern Flutter application. With the Firebase Messaging plugin, you can seamlessly integrate real-time messaging capabilities into your app, ensuring instant and reliable communication with your users.
Getting Started: A Step-by-Step Guide
Embark on your FCM journey by following these simple steps:
- Add the plugin to your pubspec.yaml file:
dependencies: firebase_messaging: ^11.0.0
- Import the plugin into your Dart code:
import 'package:firebase_messaging/firebase_messaging.dart';
- Initialize the plugin:
FirebaseMessaging messaging = FirebaseMessaging.instance;
Usage: Harnessing the Power of FCM
With the plugin initialized, you're ready to leverage FCM's full potential:
Sending Messages:
messaging.sendMessage( to: '/topics/my_topic', notification: Notification( title: 'My Title', body: 'My Body', ), );
Receiving Messages:
messaging.onMessage.listen((RemoteMessage message) { // Handle data message print('Received data message: ${message.data}'); });
Managing Notifications:
messaging.getToken().then((token) { print('FirebaseMessaging token: $token'); });
Troubleshooting: Resolving Common Issues
- "Unable to register device token" error: Ensure that you have correctly set up Firebase Core and the FCM plugin.
- "Permission denied" error: Check if your app has the necessary permissions in the AndroidManifest.xml file.
- "Failed to send FCM message" error: Verify that your API key is valid and that your Firebase project is configured correctly.