Expandable Flutter Widgets for Enhanced User Interactions
Published on by Flutter News Hub
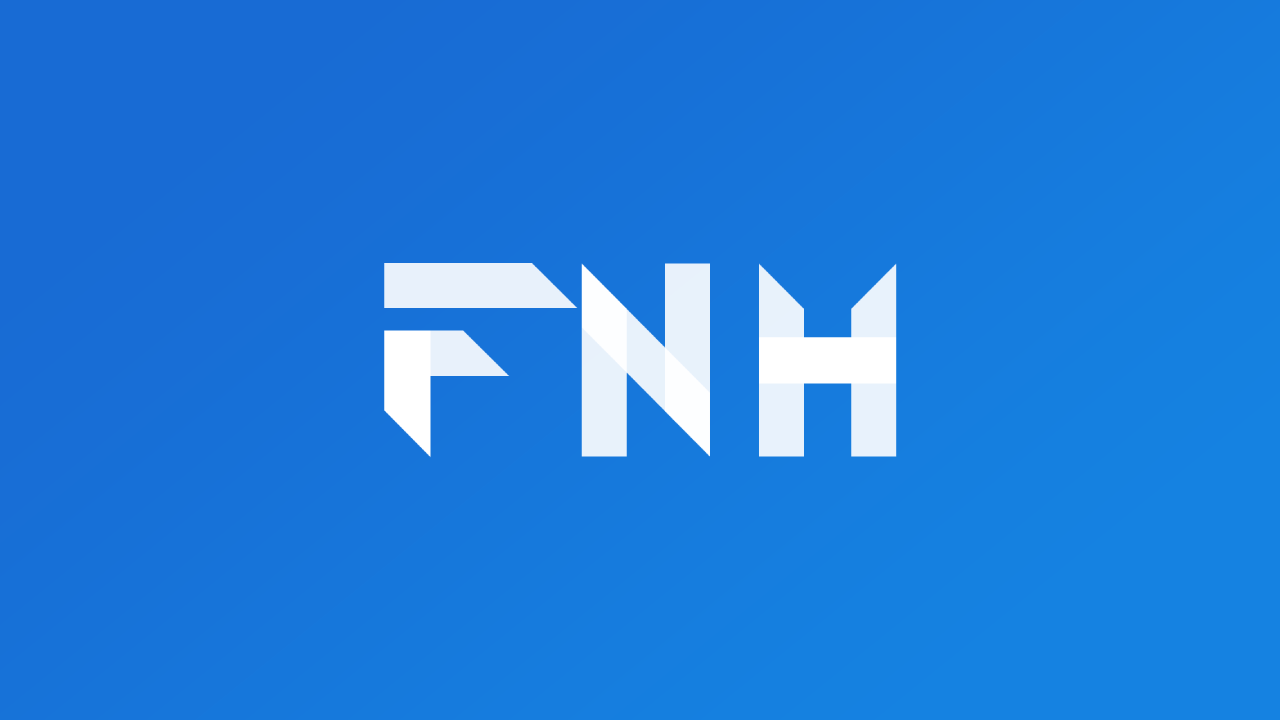
The Expandable package for Flutter enables the creation of widgets that can be expanded or collapsed by users, facilitating intuitive user interfaces that adhere to Material Design guidelines.
Usage
ExpandablePanel:
The simplest way to create an expandable widget is through ExpandablePanel:
class ArticleWidget extends StatelessWidget {
final Article article;
ArticleWidget(this.article);
@override
Widget build(BuildContext context) {
return ExpandablePanel(
header: Text(article.title),
collapsed: Text(
article.body,
softWrap: true,
maxLines: 2,
overflow: TextOverflow.ellipsis,
),
expanded: Text(
article.body,
softWrap: true,
),
tapHeaderToExpand: true,
hasIcon: true,
);
}
}
Custom Expandable Widgets:
For more customization, use a combination of Expandable, ExpandableNotifier, and ExpandableButton:
class EventPhotos extends StatelessWidget {
@override
Widget build(BuildContext context) {
return ExpandableNotifier(
child: Column(
children: [
Expandable(
collapsed: ExpandableButton(
child: buildCoverPhoto(),
),
expanded: Column(
children: [
buildAllPhotos(),
ExpandableButton(
child: Text("Back"),
),
],
),
),
],
),
);
}
}
Automatic Scrolling
Integrate ScrollOnExpand to automatically scroll to expanded widgets within a scroll view, ensuring they fit within the viewable area:
ExpandableNotifier(
child: ScrollOnExpand(
child: ExpandablePanel(
// ...
)
)
)
Themes
Expandable widgets support themes for easy visual customization and future extensibility. Themes can be set directly via constructors or through the nearest ExpandableTheme widget:
ExpandableTheme(
data: ExpandableThemeData(
iconColor: Colors.red,
animationDuration: const Duration(milliseconds: 500),
),
child: Expandable(
// 500 milliseconds animation duration
),
)
Icon Customization
Customize expand/collapse icons with:
-
hasIcon
- display icon in header -
iconSize
- icon size -
iconColor
- icon color -
iconPlacement
- icon position in header -
iconRotationAngle
- icon rotation angle -
expandIcon
- icon for collapsed state -
collapseIcon
- icon for expanded state
If using the same icon for expand/collapse, specify the same value for both expandIcon
and collapseIcon
.
Migration
For previous versions, refer to the Migration Guide: https://github.com/aryzhov/flutter-expandable/blob/master/doc/migration.md
Code Example
Expandable List of Articles:
class ArticleList extends StatelessWidget {
final List articles;
ArticleList(this.articles);
@override
Widget build(BuildContext context) {
return ListView.builder(
itemCount: articles.length,
itemBuilder: (context, index) {
return ExpandablePanel(
header: Text(articles[index].title),
collapsed: Text(
articles[index].body,
softWrap: true,
maxLines: 2,
overflow: TextOverflow.ellipsis,
),
expanded: Text(
articles[index].body,
softWrap: true,
),
);
},
);
}
}
Conclusion
The Expandable package provides a comprehensive solution for expanding and collapsing widgets, enabling developers to create interactive and engaging user interfaces that enhance the user experience.