Enhance Your Flutter Apps with TableCalendar: A Comprehensive Guide
Published on by Flutter News Hub
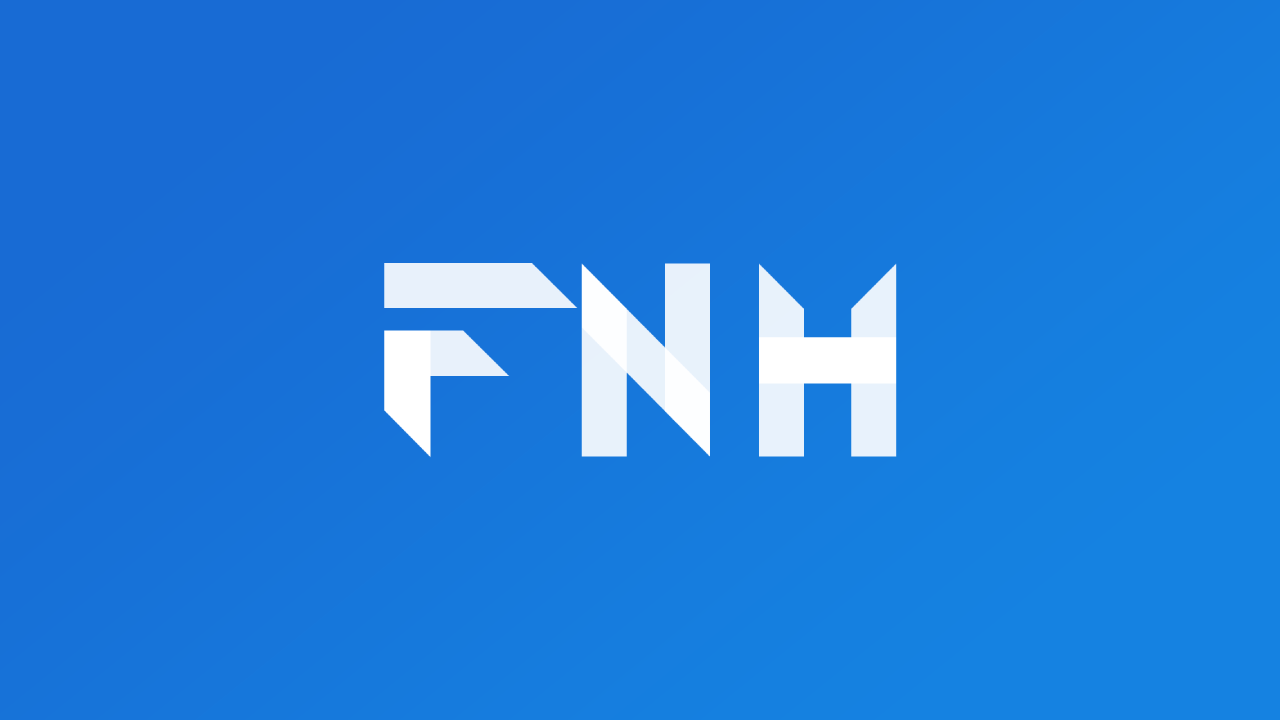
Embellish Your Calendars with TableCalendar
TableCalendar is a highly customizable calendar widget for Flutter that empowers you with a variety of features and styling options. With its user-centric design, you can easily create tailored calendar experiences for your users.
Getting Started with TableCalendar
Step 1: Installation
Add TableCalendar to your pubspec.yaml file:
dependencies: table_calendar: ^3.1.1
Step 2: Basic Setup
In your Dart code, initialize TableCalendar with essential parameters:
TableCalendar( firstDay: DateTime.utc(2010, 10, 16), lastDay: DateTime.utc(2030, 3, 14), focusedDay: DateTime.now(), );
Enriching Interactivity
Tap Interactions:
Enable user interactions by providing a callback to mark selected days:
selectedDayPredicate: (day) => isSameDay(_selectedDay, day), onDaySelected: (selectedDay, focusedDay) { setState(() { _selectedDay = selectedDay; _focusedDay = focusedDay; }); },
Format Changes:
Allow users to switch between calendar formats:
calendarFormat: _calendarFormat, onFormatChanged: (format) { setState(() { _calendarFormat = format; }); },
Managing Focused Days
To ensure the calendar reflects user interaction, update focusedDay in callbacks:
onPageChanged: (focusedDay) { _focusedDay = focusedDay; },
Adding Events to Your Calendar
Event Loading:
Provide a callback to populate events based on the provided date:
eventLoader: (day) { return _getEventsForDay(day); },
Cyclic Events:
Create repeatable events, e.g., an event on every Monday:
eventLoader: (day) { if (day.weekday == DateTime.monday) { return [Event('Cyclic event')]; } return []; },
Events on Tap:
Select events when users tap on days:
void _onDaySelected(DateTime selectedDay, DateTime focusedDay) { if (!isSameDay(_selectedDay, selectedDay)) { setState(() { _focusedDay = focusedDay; _selectedDay = selectedDay; _selectedEvents = _getEventsForDay(selectedDay); }); } }
Customizing UI with CalendarBuilders
For ultimate design flexibility, leverage CalendarBuilders:
calendarBuilders: CalendarBuilders( dowBuilder: (context, day) { if (day.weekday == DateTime.sunday) { final text = DateFormat.E().format(day); return Center( child: Text( text, style: TextStyle(color: Colors.red), ), ); } }, ),
Internationalization with Locale
Display the calendar in different languages:
TableCalendar( locale: 'pl_PL', ),
Supported Languages (with language codes):
- English (en_US)
- Polish (pl_PL)
- French (fr_FR)
- Chinese (zh_CN)
Conclusion
TableCalendar empowers you to create visually appealing and feature-rich calendars with ease. Its customization options and event handling capabilities make it ideal for a wide range of applications.