DropdownSearch: A Robust Flutter Package for Searchable Dropdowns
Published on by Flutter News Hub
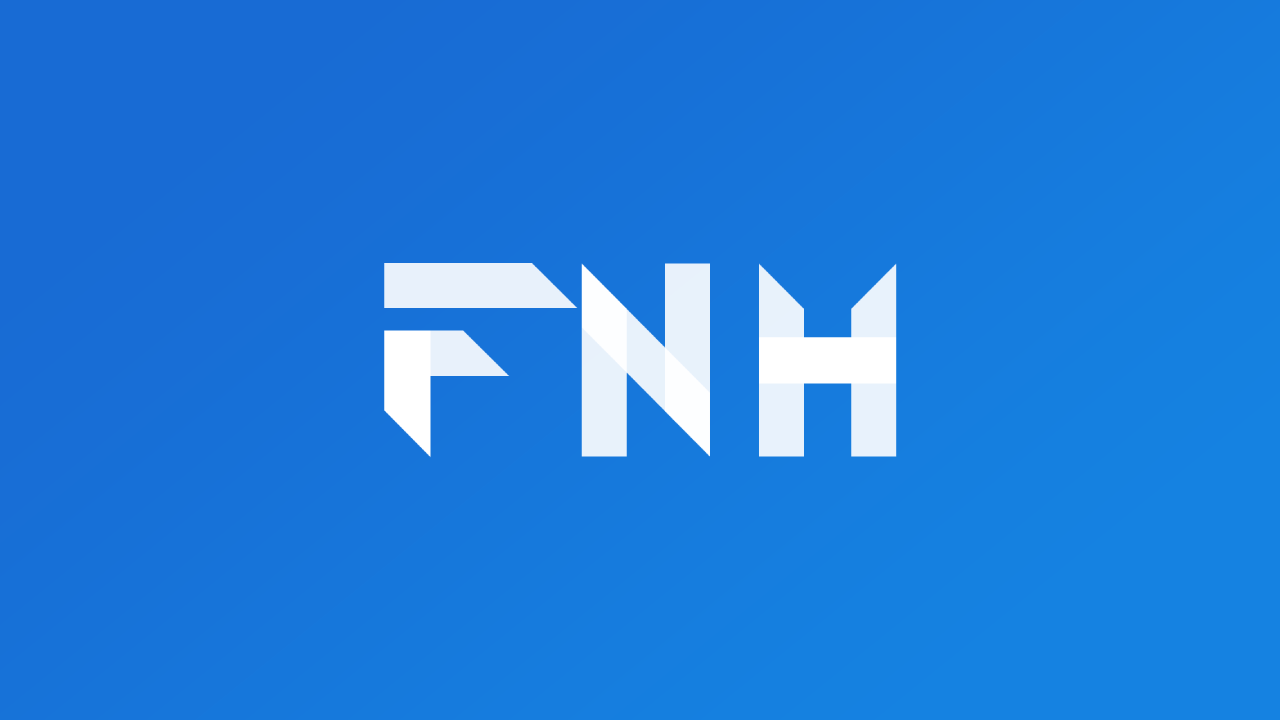
DropdownSearch is a versatile Flutter package that provides developers with a comprehensive solution for implementing searchable dropdowns in their applications. This package makes it easy to integrate customizable dropdowns with item search capabilities, making it suitable for a wide range of use cases.
Key Features
- Synchronous and Asynchronous Item Handling: Supports both offline item lists and online filtering via URLs.
- Searchable Dropdown: Allows users to search for specific items within the dropdown list.
- Multiple Dropdown Modes: Choose from menu, bottom sheet, or modal bottom sheet/dialog modes to suit your application's design.
- Single and Multi-Selection: Supports both single and multiple item selection options.
- Material Design Styling: Adheres to Material Design guidelines for a seamless user experience.
- UI Customization: Offers ample opportunities for UI customization, including item formatting and dropdown appearance.
- Light and Dark Theme Support: Automatically adapts to the device's theme settings.
- StatelessWidget Compatibility: Easy to integrate with stateless widgets.
- Multi-Level Items: Supports displaying items organized in hierarchical structures.
Simple Implementation
DropdownSearch(
popupProps: PopupProps.menu(
showSelectedItems: true,
disabledItemFn: (String s) => s.startsWith('I'),
),
items: ["Brazil", "Italia (Disabled)", "Tunisia", 'Canada'],
dropdownDecoratorProps: DropDownDecoratorProps(
dropdownSearchDecoration: InputDecoration(
labelText: "Menu mode",
hintText: "country in menu mode",
),
),
onChanged: print,
selectedItem: "Brazil",
);
Endpoint Implementation Using Dio Package
DropdownSearch(
dropdownSearchDecoration: InputDecoration(labelText: "Name"),
asyncItems: (String filter) async {
var response = await Dio().get(
"http://5d85ccfb1e61af001471bf60.mockapi.io/user",
queryParameters: {"filter": filter},
);
var models = UserModel.fromJsonList(response.data);
return models;
},
onChanged: (UserModel? data) {
print(data);
},
);
Customization
DropdownSearch provides extensive customization options, including:
- itemAsString: Customize how items are displayed in the dropdown list.
- compareFn: Define custom item comparison logic for filtering and selection.
- dropdownDecoratorProps: Set styling and appearance properties for the dropdown.
- popupProps: Configure the appearance and behavior of the popup menu.
Validation
DropdownSearch(
items: ["Brazil", "France", "Tunisia", "Canada"],
dropdownSearchDecoration: InputDecoration(labelText: "Name"),
onChanged: print,
selectedItem: "Tunisia",
validator: (String? item) {
if (item == null) return "Required field";
else if (item == "Brazil") return "Invalid item";
else return null;
},
);
Layout Customization
DropdownSearch allows you to customize the layout of dropdown items and their appearance.
DropdownSearch(
dropdownSearchDecoration: InputDecoration(labelText: "Name"),
asyncItems: (String filter) async {
// ...
},
onChanged: (UserModel? data) {
// ...
},
popupProps: PopupProps.menu(
itemBuilder: (BuildContext context, UserModel? item, bool isSelected) {
return Container(
child: Text(item.name),
);
},
),
);
Conclusion
DropdownSearch is a powerful and flexible package for creating customized and searchable dropdowns in Flutter applications. It supports a wide range of features, making it suitable for a variety of projects. Whether you need simple dropdowns or complex multi-level selections, DropdownSearch provides the tools and customization options to meet your requirements.