Displaying Local Notifications with Flutter Local Notifications Plugin
Published on by Flutter News Hub
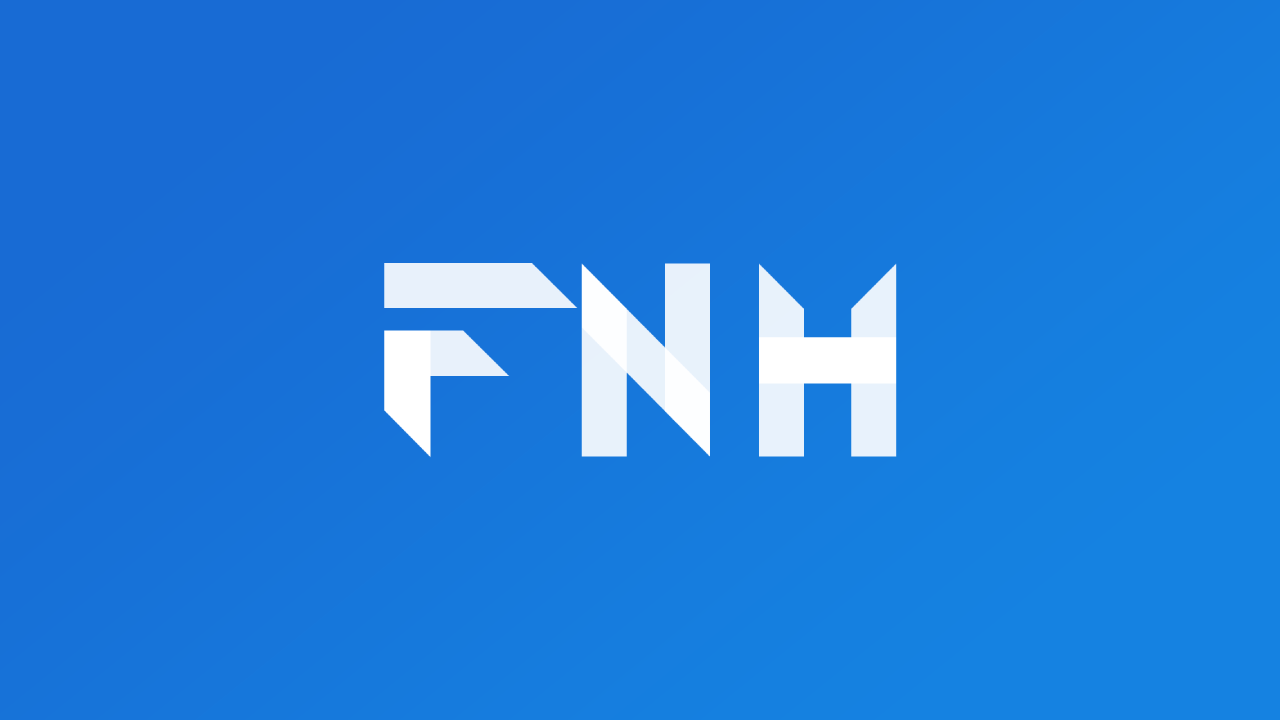
The Flutter Local Notifications Plugin allows you to display local notifications on Android, iOS, and macOS platforms.
Getting Started
1. Install the Plugin:
flutter pub add flutter_local_notifications
2. Initialize the Plugin:
import 'package:flutter_local_notifications/flutter_local_notifications.dart'; final FlutterLocalNotificationsPlugin flutterLocalNotificationsPlugin = FlutterLocalNotificationsPlugin(); /// Initialize the plugin with the desired initialization settings. /// /// In this example, we're specifying some default values for Android and iOS. /// The `onDidReceiveNotificationResponse` callback is triggered when the user clicks on a notification, and can be used to handle actions like navigation. const AndroidInitializationSettings initializationSettingsAndroid = AndroidInitializationSettings('@mipmap/app_icon'); const DarwinInitializationSettings initializationSettingsDarwin = DarwinInitializationSettings( onDidReceiveLocalNotification: (int id, String? title, String? body, String? payload) async { // Flutter will display the notification on the user's device using `payload`. // Handle notification on iOS. }, ); const LinuxInitializationSettings initializationSettingsLinux = LinuxInitializationSettings( defaultActionName: 'Open notification', ); final InitializationSettings initializationSettings = InitializationSettings( android: initializationSettingsAndroid, iOS: initializationSettingsDarwin, macOS: initializationSettingsDarwin, linux: initializationSettingsLinux, ); await flutterLocalNotificationsPlugin.initialize(initializationSettings, onDidReceiveNotificationResponse: (NotificationResponse notificationResponse) async { // Handle notification on Android. });
Displaying Notifications
1. Configure Notification Details:
const AndroidNotificationDetails androidNotificationDetails = AndroidNotificationDetails( 'channelId', 'channelName', channelDescription: 'channelDescription', importance: Importance.high, priority: Priority.high, ticker: 'ticker', ); const NotificationDetails notificationDetails = NotificationDetails(android: androidNotificationDetails);
2. Show the Notification:
await flutterLocalNotificationsPlugin.show( 0, // ID for the notification 'Notification Title', // Title of the notification 'Notification Body', // Body of the notification notificationDetails, payload: 'payload', // Optional payload to be used when the user interacts with the notification );
Scheduling Notifications
1. Calculate Local Date and Time: To schedule a notification for a specific time, you'll need to calculate the local date and time based on the desired time zone.
import 'package:timezone/data/latest_all.dart' as tz; import 'package:timezone/timezone.dart' as tz; /// Initialize the time zone database. tz.initializeTimeZones(); /// Set the local time zone. tz.setLocalLocation(tz.getLocation('America/New_York')); /// Calculate the local date and time for the notification. final localNotificationDateTime = tz.TZDateTime.now(tz.local).add(const Duration(seconds: 5));
2. Schedule the Notification:
const AndroidNotificationDetails androidNotificationDetails = AndroidNotificationDetails( 'channelId', 'channelName', channelDescription: 'channelDescription', ); const NotificationDetails notificationDetails = NotificationDetails(android: androidNotificationDetails); await flutterLocalNotificationsPlugin.zonedSchedule( 0, 'Notification Title', 'Notification Body', localNotificationDateTime, notificationDetails, androidScheduleMode: AndroidScheduleMode.exactAllowWhileIdle, uiLocalNotificationDateInterpretation: UILocalNotificationDateInterpretation.absoluteTime, );
Receiving User Input
1. Configure Notification Actions:
const DarwinNotificationAction action1 = DarwinNotificationAction.plain('id_1', 'Action 1'); const DarwinNotificationAction action2 = DarwinNotificationAction.plain('id_2', 'Action 2'); const DarwinNotificationCategory category = DarwinNotificationCategory( 'demoCategory', actions: [action1, action2], options: {DarwinNotificationCategoryOption.hiddenPreviewShowTitle}, );
2. Listen for User Actions:
/// This function will be called when the user selects a notification action. /// It can be used to handle actions like navigation or other custom logic. void notificationTapBackground(NotificationResponse notificationResponse) { // Handle user action based on the 'notificationResponse.notificationResponse.identifier' property. } /// Initialize the plugin with the notification category and action callback. await flutterLocalNotificationsPlugin.initialize( initializationSettings, onDidReceiveNotificationResponse: (NotificationResponse notificationResponse) async { // Handle notification on Android. }, onDidReceiveBackgroundNotificationResponse: notificationTapBackground, );