Discover the SlidingUpPanel: A Comprehensive Guide
Published on by Flutter News Hub
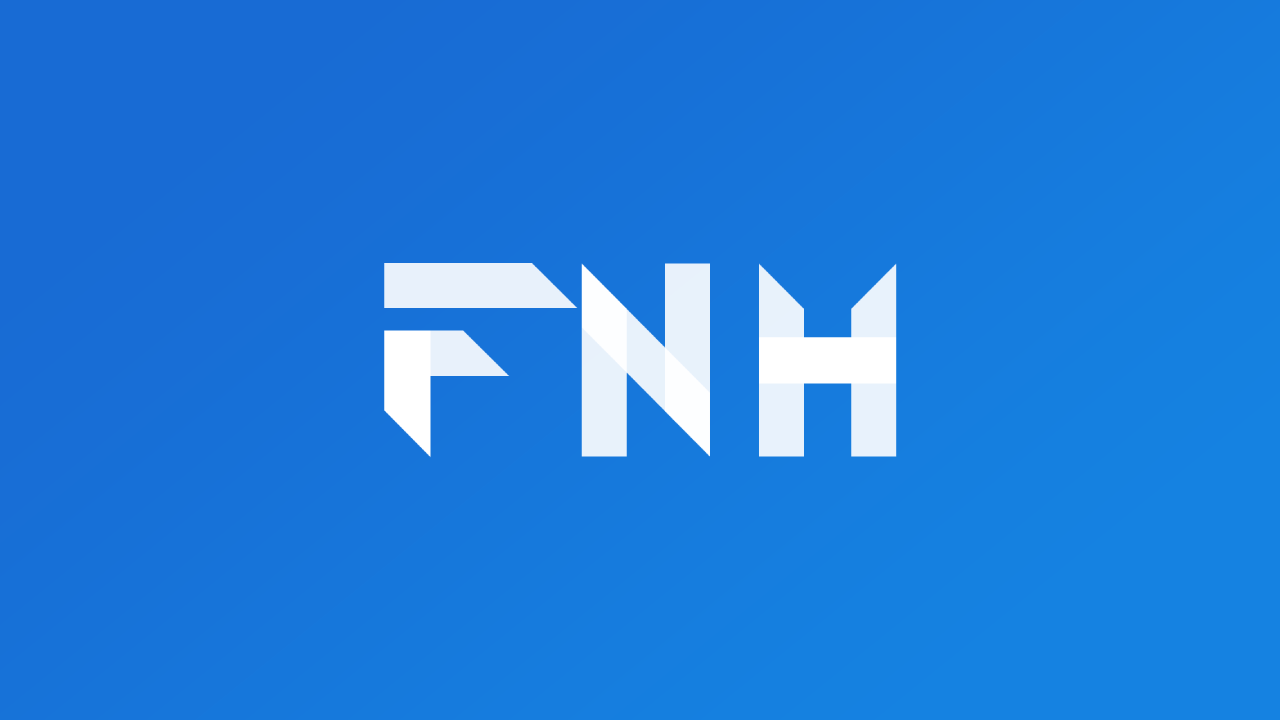
The SlidingUpPanel is a versatile Flutter widget that enables the smooth and intuitive integration of a draggable panel into your app. With its seamless transitions and customizable options, it empowers you to create engaging user experiences.
Essential Features
- Draggable Panel: The heart of the SlidingUpPanel is its draggable panel, which can be effortlessly adjusted by users.
- Customizable Panel: You have complete control over the design of the panel, allowing you to tailor it to your app's aesthetic and functionality.
- Multiple Configurations: The SlidingUpPanel offers various configurations, making it adaptable to a wide range of scenarios.
Getting Started
Including the SlidingUpPanel in your project is a breeze:
dependencies: sliding_up_panel: ^2.0.0+1
Simple Usage
There are two primary methods for using the SlidingUpPanel:
1. SlidingUpPanel as the Root Widget (Recommended)
@override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text("SlidingUpPanelExample")), body: SlidingUpPanel( panel: Center(child: Text("This is the sliding Widget")), body: Center(child: Text("This is the Widget behind the sliding panel")), ), ); }
2. Nesting the SlidingUpPanel
@override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text("SlidingUpPanelExample")), body: Stack(children: [ Center(child: Text("This is the Widget behind the sliding panel")), SlidingUpPanel( panel: Center(child: Text("This is the sliding Widget")), ) ]), ); }
Customization Options
The SlidingUpPanel provides a plethora of customization options to meet your specific requirements:
- panel: The Widget that slides into view.
- collapsed: The Widget displayed overtop the panel when collapsed.
- body: The Widget that lies underneath the sliding panel.
- header: A persistent widget that floats above the panel and attaches to its top.
- footer: A persistent widget that floats above the panel and attaches to its bottom.
- minHeight: The height of the sliding panel when fully collapsed.
- maxHeight: The height of the sliding panel when fully open.
- snapPoint: A point between minHeight and maxHeight that the panel snaps to while animating. (beta)
- border: A border to draw around the sliding panel sheet.
- borderRadius: Rounded corners for the sliding panel sheet.
- boxShadow: Shadows cast behind the sliding panel sheet.
- color: The color of the sliding panel sheet.
- padding: The amount to inset the children of the sliding panel sheet.
- margin: Empty space surrounding the sliding panel sheet.
- renderPanelSheet: Set to false to remove the sheet the panel sits upon for more advanced customization.
- panelSnapping: Enable or disable the panel's snapping behavior.
- backdropEnabled: Show a darkening shadow over the body as the panel slides open.
- backdropColor: Color of the backdrop shadow.
- backdropOpacity: Opacity of the backdrop shadow.
- backdropTapClosesPanel: Close the panel by tapping the backdrop.
- controller: Control the panel state using a PanelController.
- onPanelSlide: Callback executed as the panel slides.
- onPanelOpened: Callback executed when the panel is fully opened.
- onPanelClosed: Callback executed when the panel is fully collapsed.
- parallaxEnabled: Achieve a parallax effect as the panel slides.
- parallaxOffset: Specify the extent of the parallax effect.
- isDraggable: Toggle the user's ability to drag the panel.
- slideDirection: Choose between UP or DOWN for the panel's sliding direction.
- defaultPanelState: Open or closed state for the panel by default.
Example Customization
To enable a darkening backdrop as the panel opens:
@override Widget build(BuildContext context) { return SlidingUpPanel( backdropEnabled: true, panel: Center(child: Text("This is the sliding Widget")), body: Scaffold( appBar: AppBar(title: Text("SlidingUpPanelExample")), body: Center(child: Text("This is the Widget behind the sliding panel")), ), ); }
Advanced Techniques
1. Using the PanelController
The PanelController provides programmatic control over the panel's position and state:
PanelController _pc = new PanelController(); @override Widget build(BuildContext context) { return SlidingUpPanel( controller: _pc, panel: Center(child: Text("This is the sliding Widget")), body: _body(), ); } Widget _body() { return Container( child: Column( children: [ RaisedButton(child: Text("Open"), onPressed: () => _pc.open()), RaisedButton(child: Text("Close"), onPressed: () => _pc.close()), ], ), ); }
2. Creating Scrollable Elements in the Panel
The panel can contain scrollable elements, such as ListView:
@override Widget build(BuildContext context) { return SlidingUpPanel( panel: _scrollingList(), body: Center(child: Text("This is the Widget behind the sliding panel")), ); } Widget _scrollingList() { return ListView.builder( itemCount: 50, itemBuilder: (BuildContext context, int i) { return Container( padding: const EdgeInsets.all(12.0), child: Text("$i"), ); }, ); }
Conclusion
The SlidingUpPanel is a powerful and versatile widget that seamlessly integrates into your Flutter projects. With its customizable features and advanced capabilities, it empowers you to create engaging and intuitive user experiences.