Dio: A Comprehensive Guide to HTTP Networking for Dart/Flutter
Published on by Flutter News Hub
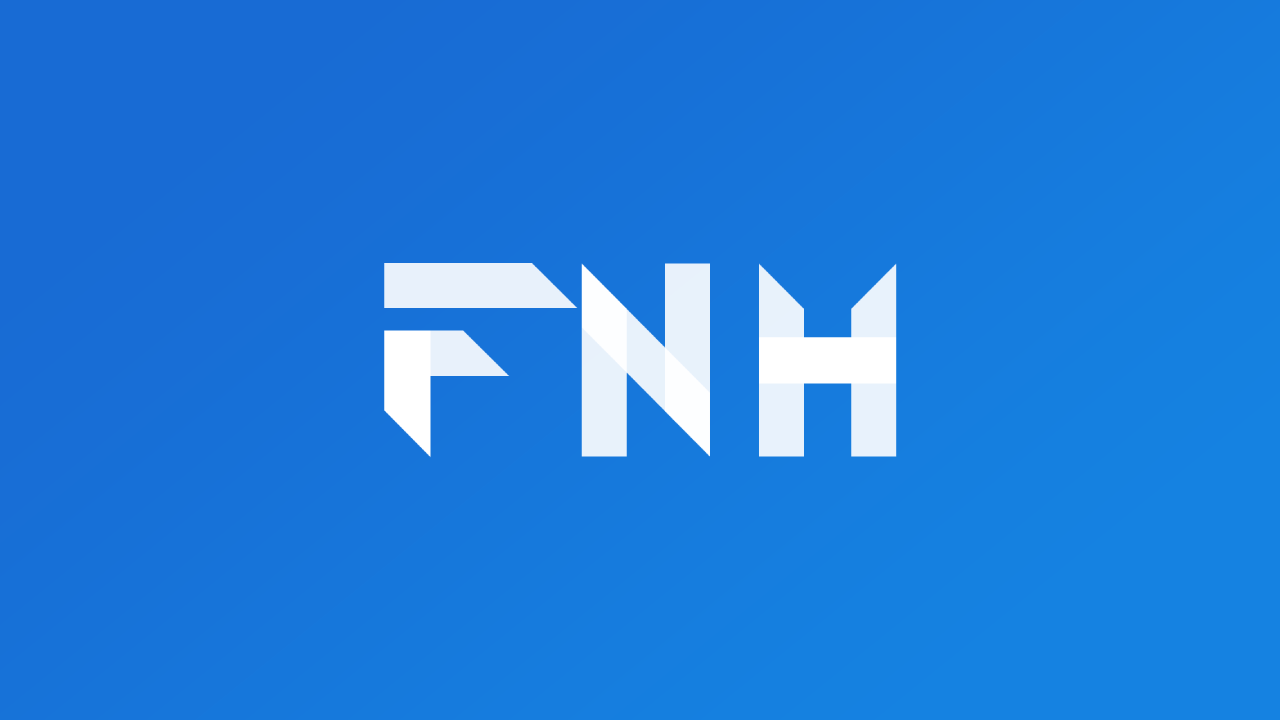
Dio is a powerful HTTP networking package for Dart and Flutter, offering a wide range of features for efficient and customizable HTTP communication. This guide provides a comprehensive overview of Dio's capabilities, including:
Getting Started
import 'package:dio/dio.dart'; final dio = Dio(); void getHttp() async { final response = await dio.get('https://dart.dev'); print(response); }
Awesome Dio
Dio is supported by a growing community of developers, as evidenced by the availability of numerous plugins and resources.
Examples
Performing a GET request:
response = await dio.get('/test');
Performing a POST request:
response = await dio.post('/test', data: {'name': 'dio'});
Sending a FormData:
final formData = FormData.fromMap({ 'name': 'dio', 'date': DateTime.now().toIso8601String(), }); final response = await dio.post('/info', data: formData);
Transformer
Transformers allow you to modify request and response data before it is sent/received.
HttpClientAdapter
HttpClientAdapter provides a bridge between Dio and HttpClient, allowing for custom HTTP configurations.
HTTP2 Support
Dio supports HTTP/2 through the dio_http2_adapter plugin.
Cancellation
Dio allows you to cancel requests using CancelTokens.
Cross-Origin Resource Sharing (CORS) on Web
CORS is supported by Dio through the addition of a CORS middleware or by modifying requests to meet the definition of simple requests.
Extending Dio Class
You can extend DioForNative or DioForBrowser to create custom Dio clients.
Additional Features
- Global configuration
- Interceptors
- Request options
- Response handling
- Error handling
- File uploading/downloading
- Timeout
- Custom adapters
Conclusion
Dio is a versatile and well-rounded HTTP networking library for Dart/Flutter. Its extensive features and ease of use make it an ideal choice for building robust and efficient applications. Whether you're developing for the web or mobile platforms, Dio provides the tools you need to handle HTTP communication effectively.