Device Preview: Preview Your Flutter App on Any Device from Your Desktop
Published on by Flutter News Hub
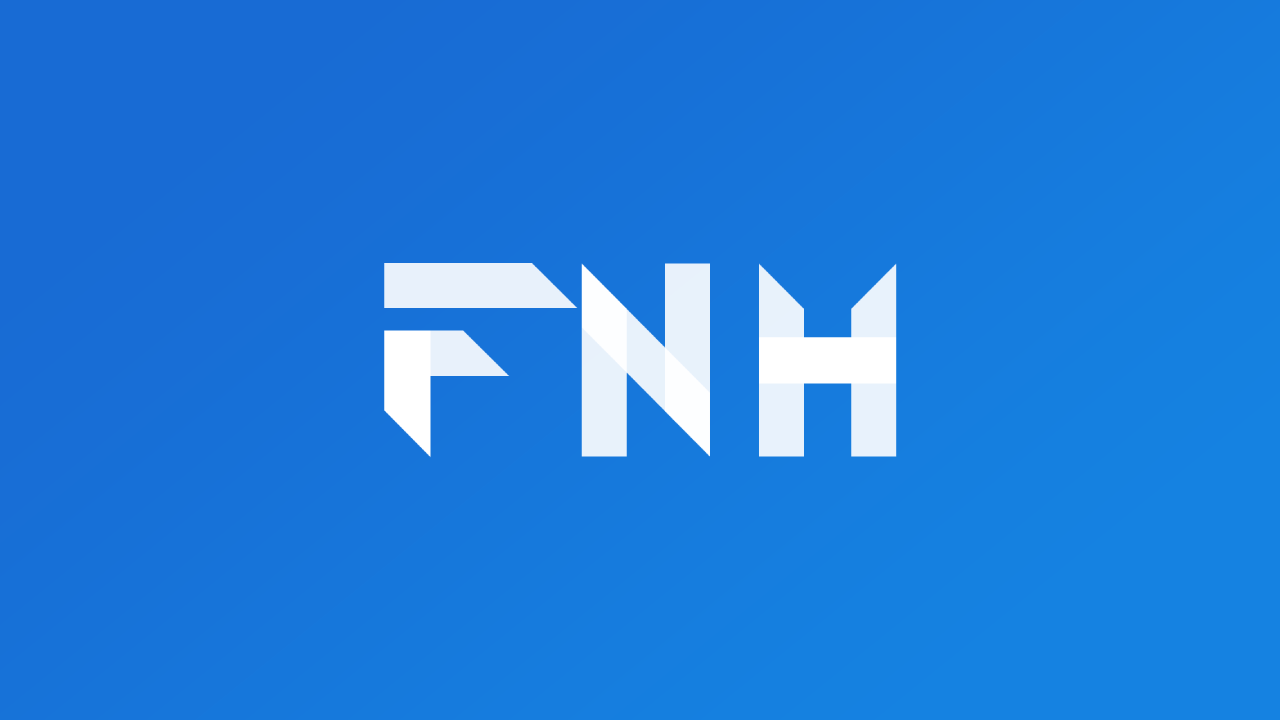
Device Preview is a powerful Flutter package that allows you to approximate how your app looks and performs on different devices, right from your desktop or laptop. This tool is ideal for developers who want to ensure their apps are optimized for a wide range of devices and screen sizes.
Key Features
- Device Emulation: Preview your app on any mobile device model, regardless of what device you're using.
- Device Orientation Control: Change the device orientation between portrait and landscape to see how your app adapts.
- System Configuration Control: Adjust system settings such as language, dark mode, text scaling, and more.
- Freeform Device Customization: Create a custom device with adjustable resolution and safe areas.
- State Persistence: Keep your application state even when switching between devices.
- Plugin System: Utilize plugins for additional functionality, such as screenshot capture or file exploration.
Setup and Usage
1. Add the Dependency
Add the Device Preview dependency to your pubspec.yaml file:
dependencies: device_preview:
2. Wrap Your App
Wrap your app's root widget with a DevicePreview widget:
import 'package:device_preview/device_preview.dart'; void main() => runApp( DevicePreview( enabled: !kReleaseMode, builder: (context) => MyApp(), ), );
3. Configure MaterialApp
Modify your MaterialApp widget to override the following properties:
class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( useInheritedMediaQuery: true, locale: DevicePreview.locale(context), builder: DevicePreview.appBuilder, theme: ThemeData.light(), darkTheme: ThemeData.dark(), home: const HomePage(), ); } }
Code Example
The following code shows how to use Device Preview with a simple Flutter app:
import 'package:device_preview/device_preview.dart'; import 'package:flutter/material.dart'; void main() => runApp( DevicePreview( enabled: !kReleaseMode, builder: (context) => MyApp(), ), ); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( useInheritedMediaQuery: true, locale: DevicePreview.locale(context), builder: DevicePreview.appBuilder, theme: ThemeData.light(), darkTheme: ThemeData.dark(), home: const HomePage(), ); } } class HomePage extends StatelessWidget { const HomePage({super.key}); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Device Preview'), ), body: Center( child: Text('This is a preview of your app!'), ), ); } }
Conclusion
Device Preview is an indispensable tool for Flutter developers. It provides a convenient and effective way to test and optimize your apps for different devices without the need for multiple physical devices. By leveraging the power of Device Preview, you can ensure that your apps deliver an exceptional user experience on any smartphone or tablet.