Dart XML: A Lightweight and Feature-Rich XML Library for Dart
Published on by Flutter News Hub
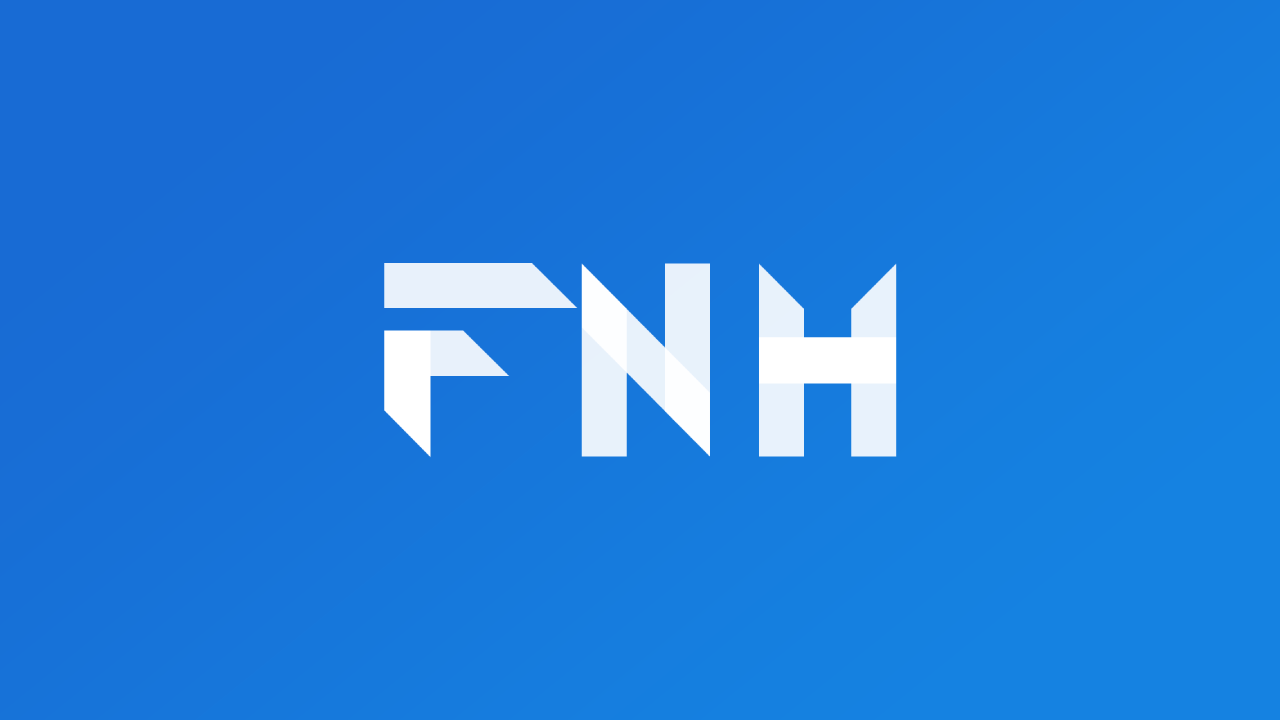
Dart XML is a robust library for working with XML documents in Dart. It offers a DOM-based object model for manipulating and querying XML documents, as well as an event-driven API for incremental reading and processing of XML streams.
Installation and Usage
To install Dart XML, follow the instructions on dart packages: https://pub.dev/packages/xml/install
Import the library into your Dart code:
import 'package:xml/xml.dart';
Reading and Writing XML
Reading XML
final bookshelfXml = '''<?xml version="1.0"?>
<bookshelf>
<book>
<title lang="en">Growing a Language</title>
<price>29.99</price>
</book>
<book>
<title lang="en">Learning XML</title>
<price>39.95</price>
</book>
<price>132.00</price>
</bookshelf>''';
final document = XmlDocument.parse(bookshelfXml);
Writing XML
print(document.toString());
print(document.toXmlString(pretty: true, indent: '\t'));
Traversing and Querying XML
Traversing XML
document.children; // direct children of the root element
document.descendants; // all descendants of the root element
document.preceding; // nodes preceding the opening tag of the current node
Querying XML
document.findAllElements('title'); // find all elements with the 'title' tag
document.xpath('/bookshelf/book[2]'); // find the second book element
Building XML
Using the Builder Pattern
final builder = XmlBuilder();
builder.element('bookshelf', nest: () {
builder.element('book', nest: () {
builder.element('title', nest: () {
builder.attribute('lang', 'en');
builder.text('Growing a Language');
});
builder.element('price', nest: 29.99);
});
builder.element('book', nest: () {
builder.element('title', nest: () {
builder.attribute('lang', 'en');
builder.text('Learning XML');
});
builder.element('price', nest: 39.95);
});
builder.element('price', nest: 132.00);
});
final document = builder.buildDocument();
Event-Driven Processing
Dart XML provides an event-based API for incremental reading and processing of XML streams.
Using Iterables
parseEvents(bookshelfXml)
.whereType<XmlTextEvent>()
.map((event) => event.value.trim())
.where((text) => text.isNotEmpty)
.forEach(print);
Using Streams
final url = Uri.parse('http://ip-api.com/xml/');
final request = await HttpClient().getUrl(url);
final response = await request.close();
await response
.transform(utf8.decoder)
.toXmlEvents()
.normalizeEvents()
.forEachEvent(onText: (event) => print(event.value));
Conclusion
Dart XML is a powerful and well-supported library for working with XML documents in Dart. Its extensive feature set and simple API make it a valuable tool for data parsing, transformation, and building.