Dart | Windows: Accessing Win32 APIs in Dart
Published on by Flutter News Hub
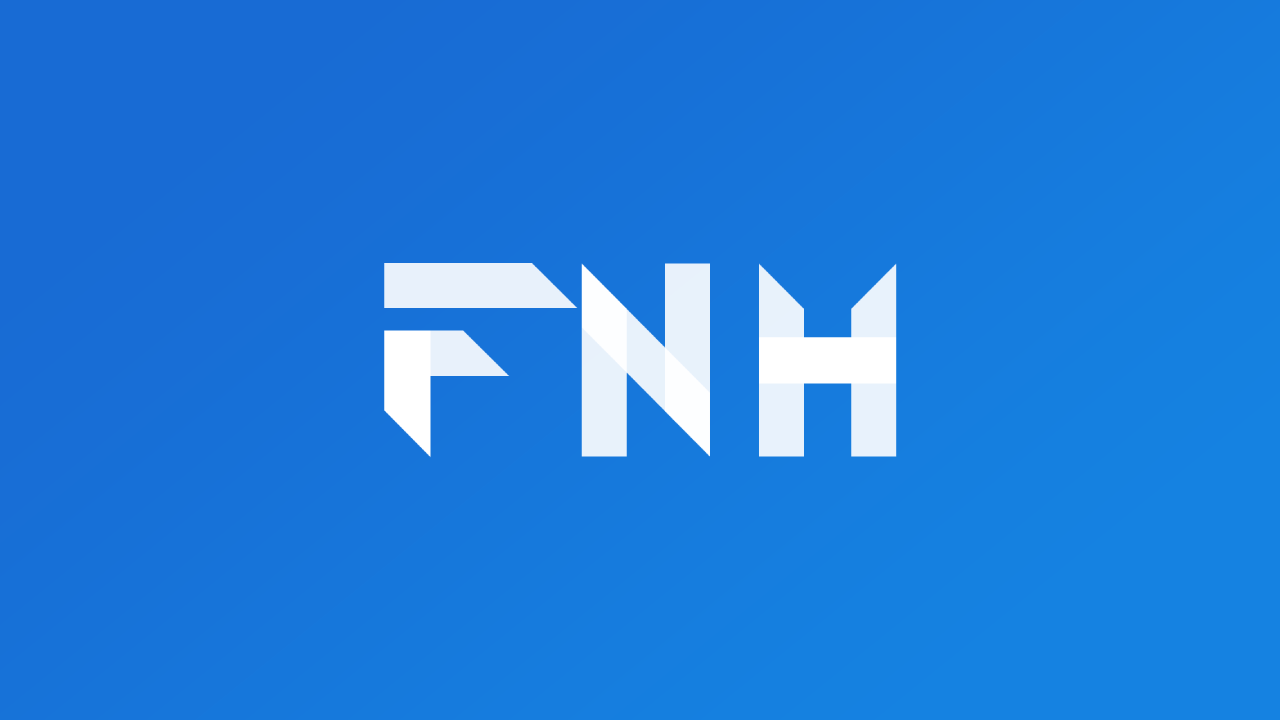
The Dart | Windows suite of packages provides access to Win32 and COM APIs, enabling Dart and Flutter apps to directly interact with the Windows operating system.
Getting Started with Win32 API Calls in Dart
To start using Win32 APIs in your Dart code, follow these steps:
- Add win32 to your pubspec.yaml:
dependencies: win32: ^latest
- Import the win32 package:
import 'package:win32/win32.dart';
Enumerating Fonts Using EnumFontFamiliesEx
To enumerate all locally-installed fonts using the EnumFontFamiliesEx API:
// Create a variable to store the font information LOGFONT lf = LOGFONT(); // Assign a callback function to be called for each font int callback(LPLOGFONT lpLogFont, TEXTMETRICW lpTextMetric, IntPtr dwType, LPARAM lParam) { print('Font: ${lpLogFont.lfFaceName}'); return 1; } // Call the EnumFontFamiliesEx API EnumFontFamiliesEx(NULL, lf, callback, LPARAM.fromInt(0));
Interacting with the Windows Command Line
You can also use the win32 package to interact with the Windows command line. Here's how to run a command:
// Create a STARTUPINFO structure STARTUPINFO si = STARTUPINFO(); si.dwFlags = STARTF_USESHOWWINDOW; si.wShowWindow = SW_HIDE; // Create a PROCESS_INFORMATION structure PROCESS_INFORMATION pi = PROCESS_INFORMATION(); // Start the process BOOL success = CreateProcess(NULL, 'cmd.exe /c dir', NULL, NULL, FALSE, CREATE_NEW_CONSOLE, NULL, NULL, si, pi); // Check if the process started successfully if (success) { // Wait for the process to finish WaitForSingleObject(pi.hProcess, INFINITE); // Close the process handle CloseHandle(pi.hProcess); }
Developing Desktop Applications
You can even develop traditional Win32 desktop applications using Dart. Here's a simple example of a window with a message-handling loop:
// Create a window HWND hwnd = CreateWindow(windowClass.name, 'Hello, Win32', WS_OVERLAPPEDWINDOW, 0, 0, 200, 100, HWND.NULL, HMENU.NULL, GetModuleHandle(NULL), NULL); // Show the window ShowWindow(hwnd, SW_SHOW); // Handle messages MSG msg; while (GetMessage(&msg, HWND.NULL, 0, 0)) { TranslateMessage(&msg); DispatchMessage(&msg); }
Conclusion
The Dart | Windows suite of packages provides a powerful bridge between Dart and the Windows operating system. By leveraging the Win32 API, Dart and Flutter developers can access a wide range of features and capabilities to create advanced and innovative applications.