Customize Your Flutter App's Splash Screen with Flutter Native Splash
Published on by Flutter News Hub
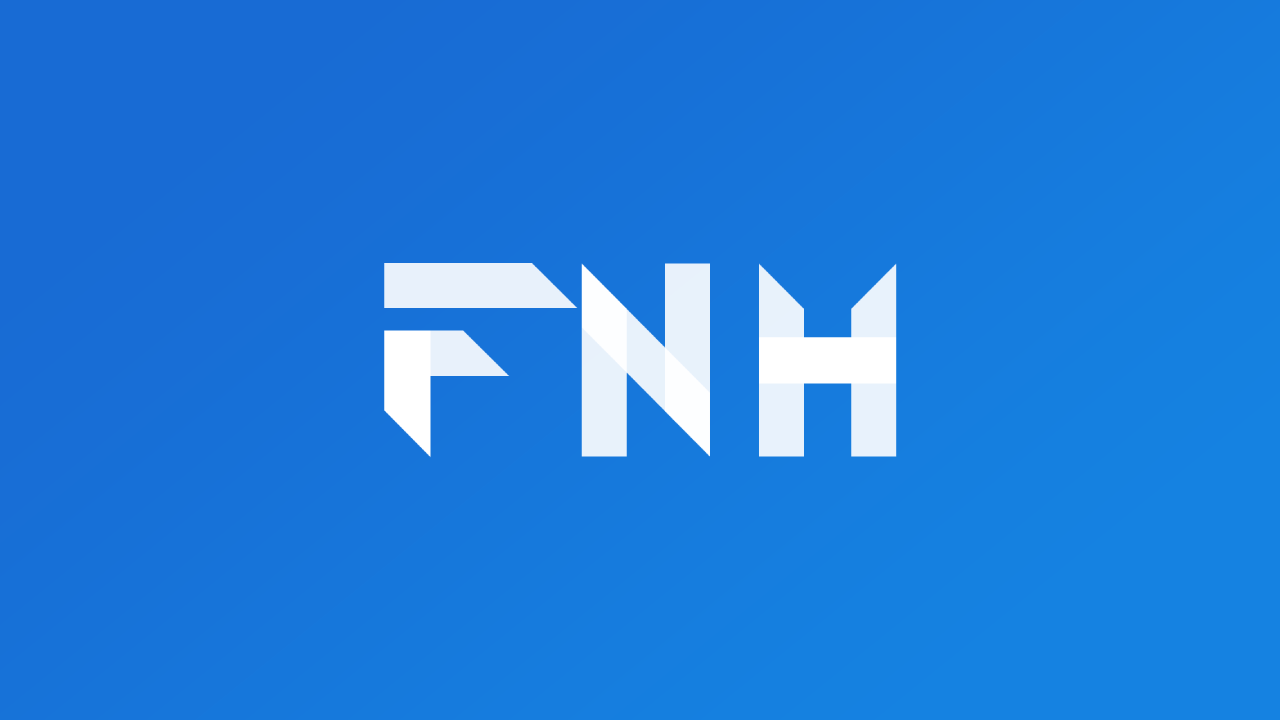
When launching a Flutter app, there's a brief period before the native app finishes loading Flutter. During this time, the native app displays a default white splash screen. The flutter_native_splash package allows you to customize this splash screen, including its background color, image, and other appearance settings.
Usage
- Add flutter_native_splash as a dependency.
dependencies: flutter_native_splash: ^2.4.0
- Set up your splash screen configuration. Create a flutter_native_splash.yaml file in your project's root folder, or add the following settings to your pubspec.yaml:
flutter_native_splash: color: "#42a5f5" image: assets/splash.png branding: assets/dart.png android_12: image: assets/android12splash.png color: "#42a5f5"
Setting Description
| color | Background color of the splash screen
| image | Background image for the splash screen
| branding | Branding image used in the splash screen
| android_12 | Specific settings for Android 12 and later
Android-Specific Settings
android_12: image: assets/android12splash.png color: "#42a5f5" icon_background_color: "#111111"
Setting Description
| image | Splash screen icon image
| color | Window background color
| icon_background_color | App icon background color
iOS-Specific Settings
ios: color: "#42a5f5" image: assets/splash-ios.png branding: assets/dart-ios.png
Setting Description
| color | Window background color
| image | Splash screen image
| branding | Branding image used in the splash screen
Web-Specific Settings
web: color: "#42a5f5" image: assets/splash-web.gif branding: assets/dart-web.gif
Setting Description
| color | Window background color
| image | Splash screen image
| branding | Branding image used in the splash screen
3. Run the package to generate the native code.
dart run flutter_native_splash:create
4. Preserve the splash screen while your app initializes (optional).
To keep the splash screen on screen while your app initializes, use the preserve() and remove() methods:
import 'package:flutter_native_splash/flutter_native_splash.dart'; void main() { WidgetsBinding widgetsBinding = WidgetsFlutterBinding.ensureInitialized(); FlutterNativeSplash.preserve(widgetsBinding: widgetsBinding); runApp(const MyApp()); } // call remove() when initialization is complete FlutterNativeSplash.remove();
Other Notes
- If you experience a white screen before the splash screen or if the splash screen was not updated correctly on iOS, run flutter clean and recompile your app.
- This package modifies files on Android (launch_background.xml, styles.xml), iOS (LaunchScreen.storyboard, Info.plist), and Web (index.html). If you have manually modified these files, this plugin may not work properly.