Creating Customizable Timelines with TimelineTile
Published on by Flutter News Hub
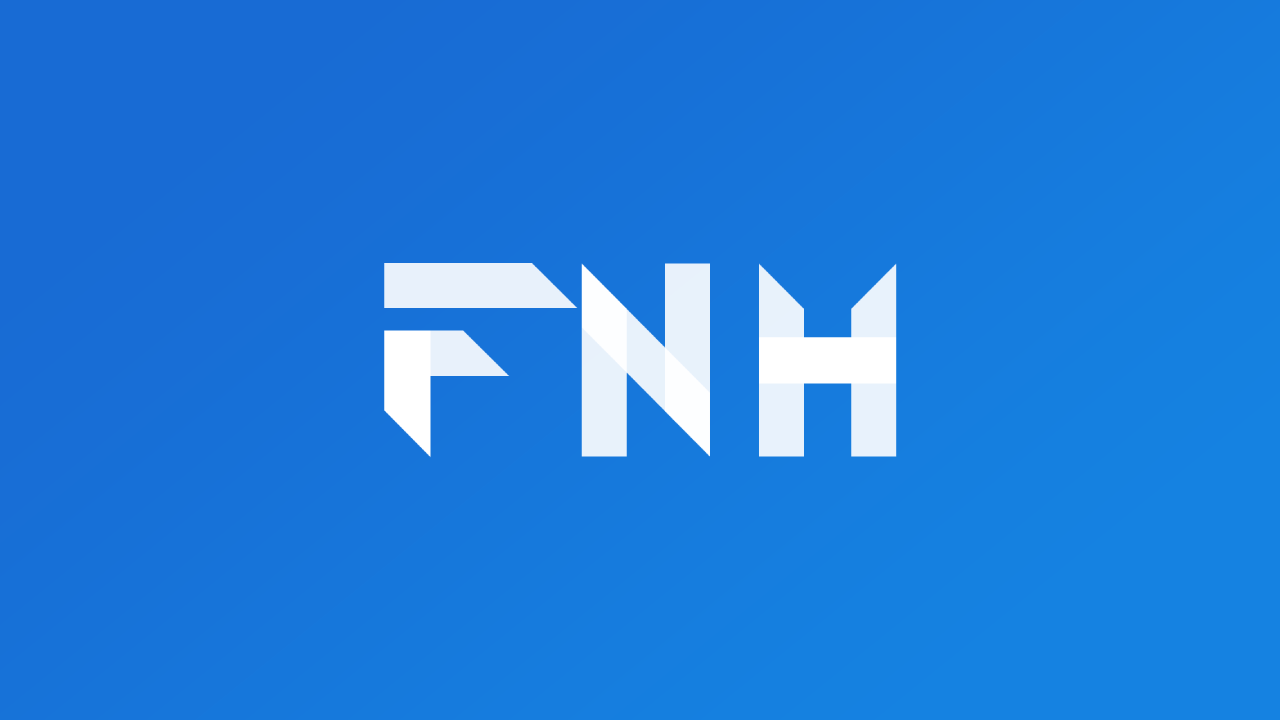
Building sophisticated timelines in Flutter is now easier than ever with the TimelineTile package. This comprehensive guide will walk you through the fundamentals of TimelineTile, empowering you to create visually compelling and informative timelines for your applications.
Getting Started
To get started with TimelineTile, simply import the package into your project:
import 'package:timeline_tile/timeline_tile.dart';
A Timeline tile can be created with the following code:
TimelineTile()
This will generate a default vertical timeline tile aligned to the start with a height of 100.
Customizing Alignment
TimelineTile supports four alignment options:
- TimelineAlign.start: Aligns the tile to the start
- TimelineAlign.end: Aligns the tile to the end
- TimelineAlign.center: Aligns the tile to the center
- TimelineAlign.manual: Allows you to manually specify the alignment using lineXY (more on this later)
Manual Indicator Alignment
With TimelineAlign.manual, you can precisely position the indicator within the tile using lineXY. This value ranges from 0.0 to 1.0, representing a percentage of the tile's size. For example:
TimelineTile( alignment: TimelineAlign.manual, lineXY: 0.3, ... )
This will align the indicator 30% of the way from the start of the tile.
First and Last Tiles
You can specify whether a tile is the first or last in a timeline, which determines the visibility of the "before" and "after" lines.
TimelineTile( isFirst: true, isLast: true, ... )
Creating a Timeline
To create a timeline, simply combine multiple TimelineTile widgets. You can control whether an indicator is displayed with the hasIndicator flag:
ListView( children: [ TimelineTile( ... ), TimelineTile( ... ), ... ], )
Customizing the Indicator
The default indicator is a circle, but you can customize it with the IndicatorStyle class. This allows you to set the color, position, padding, and even add an icon:
TimelineTile( indicatorStyle: IndicatorStyle( color: Colors.blue, icon: Icon(Icons.check), iconSize: 20, ... ), ... )
Custom Indicator
Alternatively, you can provide your custom indicator component with the indicator parameter. However, you must manage its size with the width and height parameters:
TimelineTile( indicator: MyCustomIndicator(), width: 50, height: 50, ... )
Customizing the Lines
The LineStyle class allows you to customize both the "before" and "after" lines. You can set their color, thickness, and even add a gradient:
TimelineTile( lineStyle: LineStyle( color: Colors.grey, thickness: 2, ... ), ... )
Connecting Tiles with TimelineDivider
TimelineDivider enables you to connect tiles that are aligned differently using TimelineAlign.manual. This is particularly useful for creating complex timelines:
TimelineTile( lineXY: 0.3, ... ), TimelineDivider(), TimelineTile( axis: TimelineAxis.horizontal, lineXY: 0.7, ... ),
Conclusion
TimelineTile offers a powerful and intuitive way to build customizable timelines in Flutter. With its extensive customization options and support for manual alignment, you can create visually stunning and informative timelines that meet your exact specifications. Whether you're building a simple timeline or a complex tracking system, TimelineTile has you covered. Get started today and elevate your timelines to the next level!