Create Stunning PDFs with Dart/Flutter: A Comprehensive Guide
Published on by Flutter News Hub
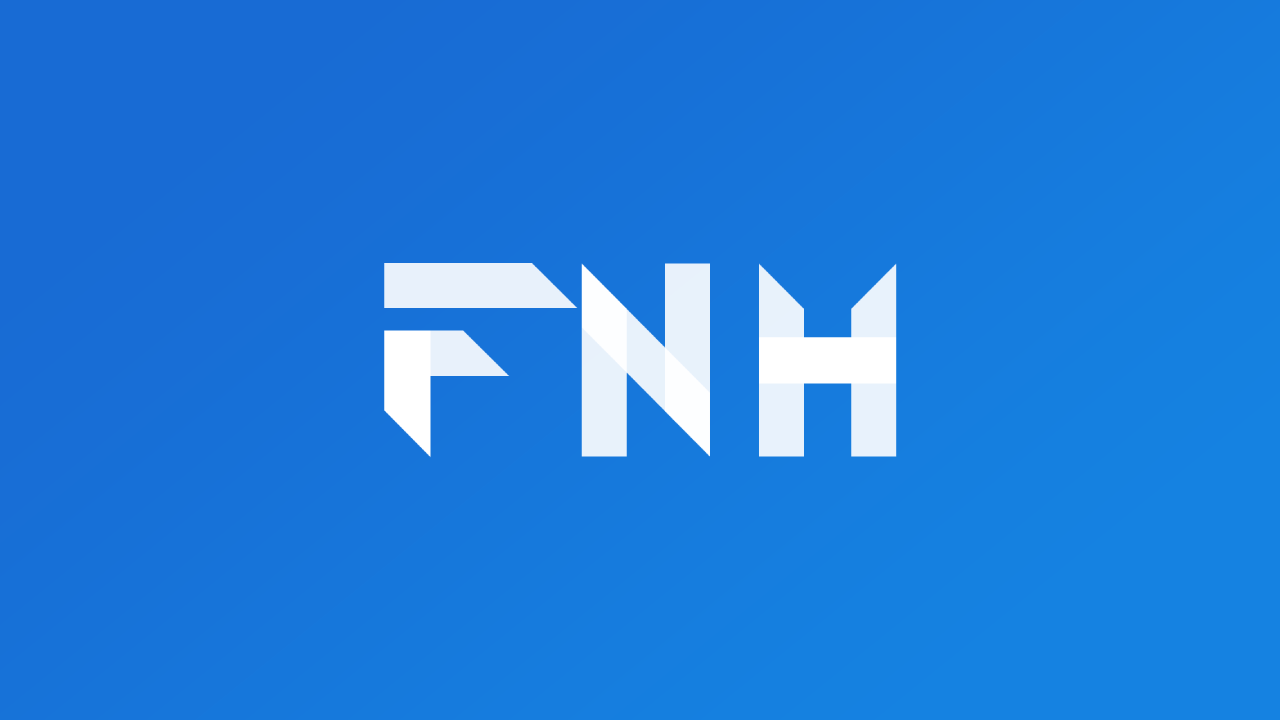
Harness the power of the Pdf library in Dart/Flutter to effortlessly generate high-quality multi-page PDF documents. This versatile library boasts an easy-to-use widget system, coupled with meticulous PDF bit generation, enabling you to create custom PDFs with graphics, images, and text.
Getting Started
Install the Pdf Library:
Add the following dependency to your pubspec.yaml file:
dependencies: pdf: ^latest
Import the libraries:
import 'package:pdf/pdf.dart'; import 'package:pdf/widgets.dart' as pw;
Create a New PDF Document:
final pdf = pw.Document();
Add a Page to the Document:
pdf.addPage(pw.Page( pageFormat: PdfPageFormat.a4, build: (pw.Context context) { return pw.Center(child: pw.Text("Hello World")); }, ));
Adding Images and SVGs
Load an Image from a File (Mobile):
final image = pw.MemoryImage(File('test.webp').readAsBytesSync()); pdf.addPage(pw.Page( build: (pw.Context context) { return pw.Center(child: pw.Image(image)); }, ));
Load an Image from an Asset File (Web):
Convert the image to a Uint8List:
final img = await rootBundle.load('assets/images/logo.jpg'); final imageBytes = img.buffer.asUint8List();
Create an image from the Uint8List:
pw.Image image1 = pw.Image(pw.MemoryImage(imageBytes));
Implement the image in a container:
pw.Container( alignment: pw.Alignment.center, height: 200, child: image1, );
Load an SVG:
String svgRaw = ''' <svg viewBox="0 0 50 50" xmlns="http://www.w3.org/2000/svg"> <ellipse style="fill: grey; stroke: black;" cx="25" cy="25" rx="20" ry="20"></ellipse> </svg> '''; final svgImage = pw.SvgImage(svg: svgRaw); pdf.addPage(pw.Page( build: (pw.Context context) { return pw.Center(child: svgImage); }, ));
Using TrueType Fonts and Emojis
Use a TrueType Font:
final Uint8List fontData = File('open-sans.ttf').readAsBytesSync(); final ttf = pw.Font.ttf(fontData.buffer.asByteData()); pdf.addPage(pw.Page( pageFormat: PdfPageFormat.a4, build: (pw.Context context) { return pw.Center( child: pw.Text('Hello World', style: pw.TextStyle(font: ttf, fontSize: 40)), ); }, ));
Use Google Fonts:
final font = await PdfGoogleFonts.nunitoExtraLight(); pdf.addPage(pw.Page( pageFormat: PdfPageFormat.a4, build: (pw.Context context) { return pw.Center( child: pw.Text('Hello World', style: pw.TextStyle(font: font, fontSize: 40)), ); }, ));
Display Emojis:
final emoji = await PdfGoogleFonts.notoColorEmoji(); pdf.addPage(pw.Page( pageFormat: PdfPageFormat.a4, build: (pw.Context context) { return pw.Center( child: pw.Text( 'Hello 🐒💁👌🎍😍🦊👨 world!', style: pw.TextStyle(fontFallback: [emoji], fontSize: 25)), ); }, ));
Saving the PDF File
Save the PDF File (Mobile):
final file = File('example.pdf'); await file.writeAsBytes(await pdf.save());
Save the PDF File (Web):
var savedFile = await pdf.save(); List fileInts = List.from(savedFile); html.AnchorElement(href: "data:application/octet-stream;charset=utf-16le;base64,$") ..setAttribute("download", "example.pdf") ..click();
Encryption, Digital Signature, and Loading an Existing PDF
Enhance PDF security with encryption using RC4-40, RC4-128, AES-128, and AES-256. Implement Digital Signature using SHA1 or SHA-256 with your x509 certificate. Utilize the PDF parser to load existing documents, modify pages, and apply signatures. For more details, visit the pdf_crypto library documentation: https://pub.nfet.net/pdf_crypto/
Conclusion
Elevate your PDF creation capabilities with the Pdf library for Dart/Flutter. Its ease of use and comprehensive features empower you to generate professional-quality documents for various applications, from mobile to web.