Create Custom Notifications with Awesome Notifications
Published on by Flutter News Hub
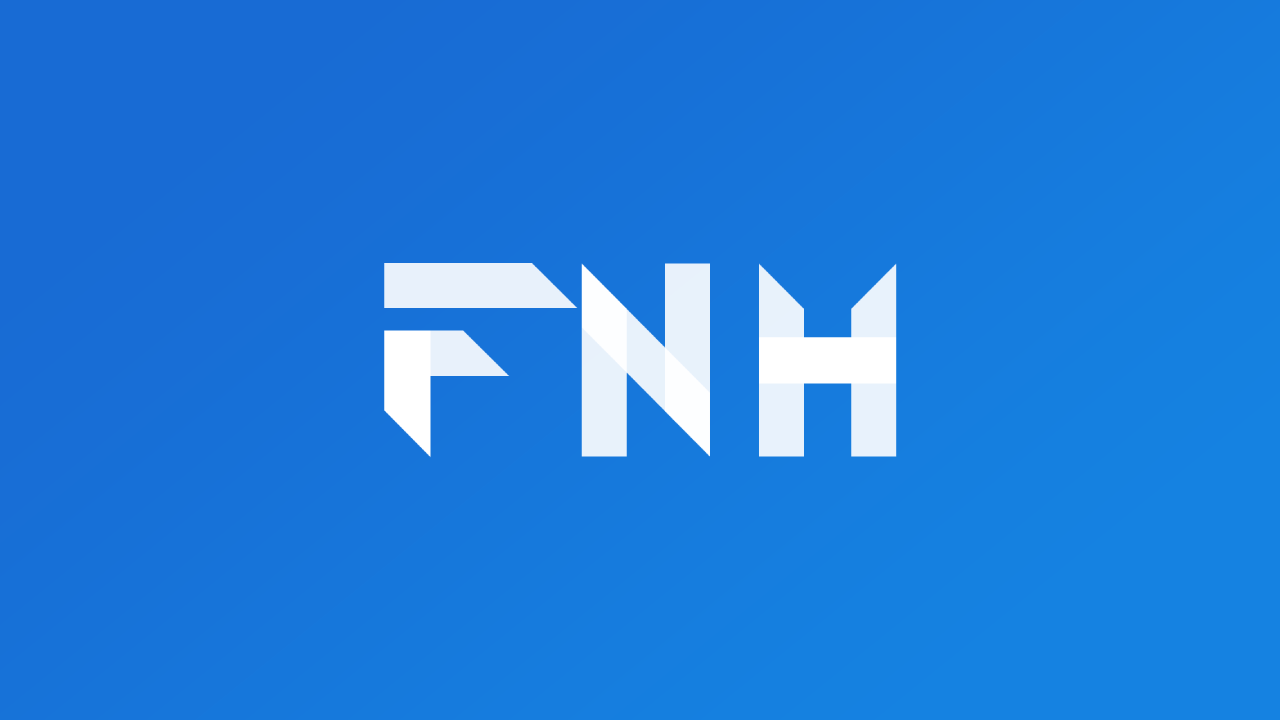
Awesome Notifications is a Flutter plugin that allows you to create and customize local and push notifications on Android and iOS. It offers a wide range of features, including customizable layouts, action buttons, and schedules.
Getting Started
To use Awesome Notifications, first add the following dependency to your pubspec.yaml file:
dependencies: awesome_notifications: ^0.9.0
Import the plugin package into your Dart code:
import 'package:awesome_notifications/awesome_notifications.dart';
Initialize the plugin with at least one native icon and one channel:
AwesomeNotifications().initialize( // set the icon to null if you want to use the default app icon 'resource://drawable/res_app_icon', [ NotificationChannel( channelGroupKey: 'basic_channel_group', channelKey: 'basic_channel', channelName: 'Basic notifications', channelDescription: 'Notification channel for basic tests', defaultColor: Color(0xFF9D50DD), ledColor: Colors.white) ], // Channel groups are only visual and are not required channelKey: 'basic_channel_group', channelGroupName: 'Basic group') debug: true );
Creating Local Notifications
To create a local notification, use the createNotification method:
AwesomeNotifications().createNotification( content: NotificationContent( id: 10, channelKey: 'basic_channel', actionType: ActionType.Default, title: 'Hello World!', body: 'This is my first notification!', ), );
Customizing Notifications
You can customize notifications by specifying various parameters, such as:
- Channel Key: Group notifications into channels with different properties.
- Action Type: Choose from several action types, such as Default, SilentAction, and SilentBackgroundAction.
- Layout Type: Use pre-defined layout types or create your own.
- Media Source Type: Display images or media from different sources, such as assets, networks, or files.
- Notification Importance: Set the importance level of the notification.
- Wake Up Screen: Wake up the device screen even when locked.
- Full Screen Intent: Display the notification in full screen mode.
Refer to the Awesome Notifications documentation for a complete list of customization options.
Scheduling Notifications
You can schedule notifications to be displayed at specific times or intervals. Awesome Notifications supports three types of schedules:
- NotificationInterval: Schedule notifications at a regular interval.
- NotificationCalendar: Schedule notifications based on a specific date and time.
- NotificationAndroidCrontab: Schedule notifications based on a Cron expression (Android only).
Handling Notification Events
Listen for notification events to capture user interactions with notifications, such as clicking action buttons or dismissing the notification.
AwesomeNotifications().setListeners( onActionReceivedMethod: (ReceivedAction receivedAction){ // Your code goes here }, onNotificationCreatedMethod: (ReceivedNotification receivedNotification){ // Your code goes here }, onNotificationDisplayedMethod: (ReceivedNotification receivedNotification){ // Your code goes here }, onDismissActionReceivedMethod: (ReceivedAction receivedAction){ // Your code goes here }, );
Additional Android Features
The Android foreground service feature allows you to display notifications as foreground services. This is necessary for certain notification types, such as alarms, full-screen intents, and background task progress.
import 'package:awesome_notifications/android_foreground_service.dart';
Add the following to your AndroidManifest.xml file:
<uses-permission android:name="android.permission.FOREGROUND_SERVICE" /> <service android:name="me.carda.awesome_notifications.core.services.ForegroundService" android:enabled="true" android:exported="false" android:stopWithTask="true" android:foregroundServiceType=AllServiceTypesThatYouChosen ></service>
To create a notification as a foreground service, use the startForeground method:
AndroidForegroundService.startAndroidForegroundService( foregroundStartMode: ForegroundStartMode.stick, foregroundServiceType: ForegroundServiceType.phoneCall, content: NotificationContent( id: 2341234, body: 'Service is running!', title: 'Android Foreground Service', channelKey: 'basic_channel', bigPicture: 'asset://assets/images/android-bg-worker.jpg', notificationLayout: NotificationLayout.BigPicture, category: NotificationCategory.Service, ), actionButtons: [ NotificationActionButton(key: 'SHOW_SERVICE_DETAILS', label: 'Show details') ], );
Conclusion
Awesome Notifications provides a powerful and flexible way to create and customize local and push notifications on Android and iOS. Its extensive feature set allows developers to create engaging and informative notifications that meet their specific needs.