Convex Bottom Bar: A Custom Bottom Navigation Bar for Flutter
Published on by Flutter News Hub
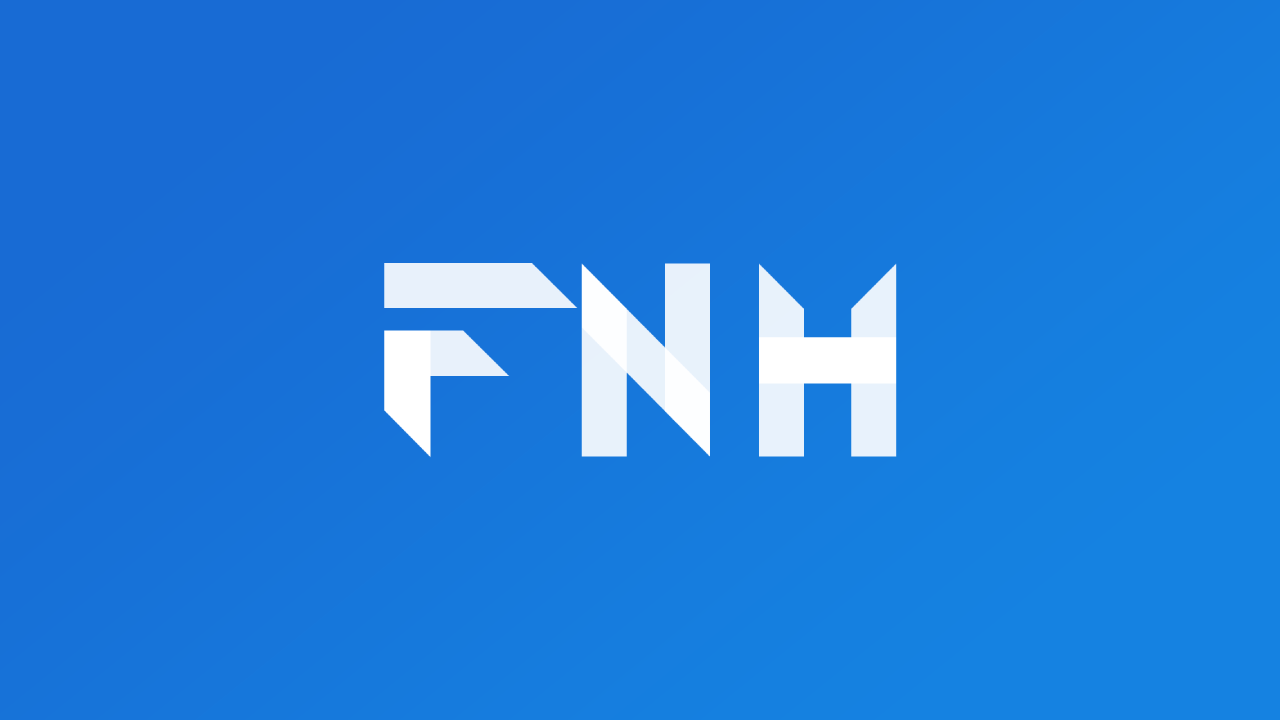
The official BottomAppBar in Flutter only allows for a notch FAB with an app bar, but sometimes a convex FAB is needed. The ConvexAppBar is an alternative bottom navigation bar inspired by the BottomAppBar and NotchShape implementations, featuring a convex FAB.
Key Features
- Multiple internal styles
- Customizable app bar theme
- Builder API for creating custom styles
- Badge support on tab menu
- Elegant transition animations
- Style hook API for overriding internal styles
- RTL support
Usage
To use ConvexAppBar, typically you can set it as the bottomNavigationBar of a Scaffold.
import 'package:convex_bottom_bar/convex_bottom_bar.dart'; Scaffold( bottomNavigationBar: ConvexAppBar( items: [ TabItem(icon: Icons.home, title: 'Home'), TabItem(icon: Icons.map, title: 'Discovery'), TabItem(icon: Icons.add, title: 'Add'), TabItem(icon: Icons.message, title: 'Message'), TabItem(icon: Icons.people, title: 'Profile'), ], onTap: (int i) => print('click index=$i'), ) );
Theming
The app bar uses a default style, but you can customize it using the following attributes:
- backgroundColor
- gradient
- height
- color
- activeColor
- curveSize
- top
- cornerRadius
- style
- chipBuilder
Badge
To add a badge to a tab, use the ConvexAppBar.badge method. It accepts an array of badges, where each badge value can be a String, IconData, Color, or Widget.
ConvexAppBar.badge( badges: { 0: '1', 2: Icon(Icons.add), 4: Colors.red, }, items: [...], onTap: (int i) => print('click index=$i'), );
Single Button
If you only need a single button, you can use the ConvexButton.fab.
Scaffold( appBar: AppBar(title: const Text('ConvexButton Example')), body: Center(child: Text('count $count')), bottomNavigationBar: ConvexButton.fab( onTap: () => setState(() => count++), ), );
Style Hook
The style hook allows you to update the tab style without defining a new tab style.
StyleProvider( style: Style(), child: ConvexAppBar( style: TabStyle.fixedCircle, items: [...], backgroundColor: _tabBackgroundColor, ) ) class Style extends StyleHook { @override double get activeIconSize => 40; @override double get activeIconMargin => 10; @override double get iconSize => 20; @override TextStyle textStyle(Color color) => TextStyle(fontSize: 20, color: color); }
RTL Support
RTL is supported internally and should work fine when usingTextDirection.
Directionality( textDirection: TextDirection.rtl, child: Scaffold(body: ConvexAppBar(/*TODO ...*/)), )
Custom Example
To create a fully custom tab, use the ConvexAppBar.builder.
Scaffold( bottomNavigationBar: ConvexAppBar.builder( count: 5, backgroundColor: Colors.blue, itemBuilder: Builder(), ) ); // user defined class class Builder extends DelegateBuilder { @override Widget build(BuildContext context, int index, bool active) { return Text('TAB $index'); } }
Documentation
For more detailed documentation and examples, please refer to the official documentation: