Comprehensive Guide to Internationalization and Localization with Flutter's Intl Package
Published on by Flutter News Hub
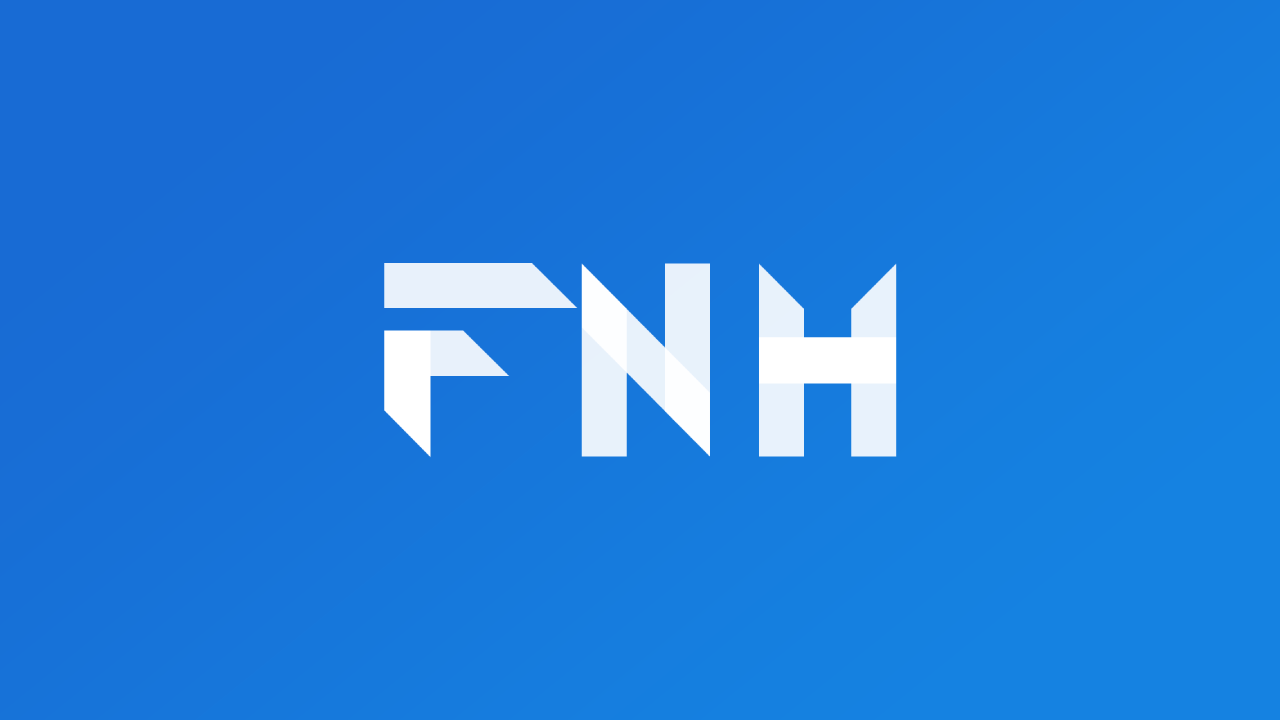
Flutter's intl package provides a robust set of tools for implementing internationalization and localization (i18n/l10n) in your Flutter applications. This allows you to support multiple languages, adapt content to different locales, and provide culturally sensitive experiences for your users.
Getting Started
1. Import the intl Package:
import 'package:intl/intl.dart';
2. Initialize the Default Locale:
By default, intl uses the system locale. To set a custom default locale:
Intl.defaultLocale = 'fr_CA';
Message Translation
1. Wrap Messages with Intl.message:
Use Intl.message to wrap messages that need to be translated. Provide a name, arguments (if any), and a description for translators.
String hiMessage() => Intl.message('Hi there!', name: 'hiMessage', args: [], desc: 'A friendly greeting');
2. Extract Messages for Translation:
Run extract_to_arb.dart to create an intl_messages.arb file containing all translatable messages.
$ pub run intl_translation:extract_to_arb --output-dir=target/directory my_app.dart
3. Generate Translated Libraries:
Translate the intl_messages.arb file and run generate_from_arb.dart to create localized libraries.
$ pub run intl_translation:generate_from_arb --generated_file_prefix=my_app_messages <translated_ARB_files>
4. Load Localized Messages:
Import the generated library and initialize it for the desired locale.
import 'my_app_messages_all.dart'; initializeMessages('fr').then((_) => print(hiMessage()));
Number Formatting
1. Create a NumberFormat Instance:
Specify a format string and optional locale.
var numberFormat = NumberFormat('###.0#', 'en_US'); print(numberFormat.format(12.345)); // Output: 12.35
2. Use Number Symbols:
Access the number symbol data for the current locale.
print(numberFormat.symbols.DECIMAL_SEP); // Output: '.' (decimal separator)
Date Formatting
1. Create a DateFormat Instance:
Use a skeleton or an explicit pattern to create a date formatter.
DateFormat dateFormatter = DateFormat.yMMMMEEEEd('en_US'); print(dateFormatter.format(DateTime.now())); // Output: 'Wednesday, January 10, 2012'
2. Parse Dates:
Parse a date string using the same skeleton or pattern.
DateFormat dateParser = DateFormat.yMd('en_US'); DateTime date = dateParser.parse('1/10/2012'); // Date object representing January 10, 2012
Bidirectional Text
1. Create a BidiFormatter:
Specify directionality with BidiFormatter.RTL or BidiFormatter.LTR.
BidiFormatter bidiFormatter = BidiFormatter.RTL(); String wrappedText = bidiFormatter.wrap('Hello world!');
2. Wrap Text:
Wrap the text with directional indicator characters or HTML spans.
print(bidiFormatter.wrapWithUnicode('Hello world!')); // Output: 'Hello world!'\u202C (RTL mark) print(bidiFormatter.wrapWithSpan('Hello world!')); // Output: '<span dir="rtl">Hello world!</span>'
Conclusion
The intl package provides a powerful and comprehensive set of features for internationalization and localization. By using its capabilities, you can deliver language-aware and culturally sensitive experiences to your Flutter users.