Capture and Save Widget Screenshots with Ease
Published on by Flutter News Hub
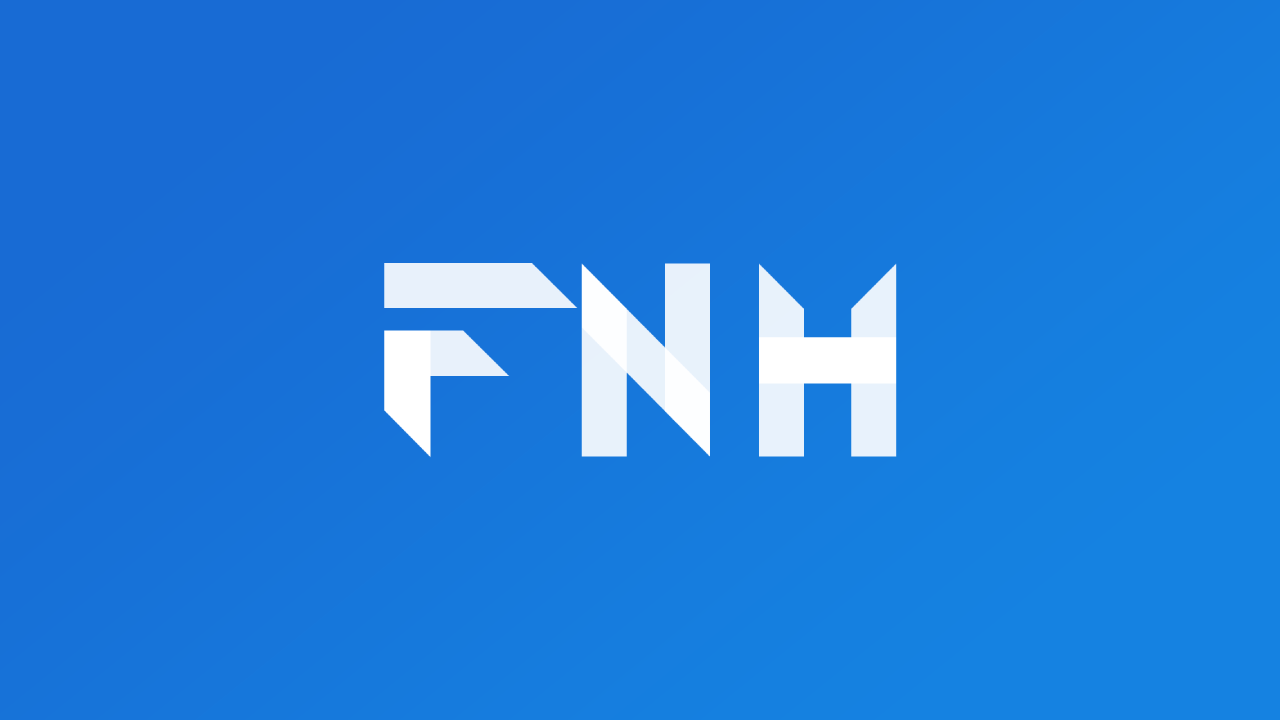
The "Screenshot" package empowers you to effortlessly capture images of your Flutter widgets. Whether you need to take full-screen screenshots or capture individual widgets like text, this package has you covered.
1. Getting Started:
import 'package:screenshot/screenshot.dart'; class MyHomePage extends StatelessWidget { final ScreenshotController screenshotController = ScreenshotController(); @override Widget build(BuildContext context) { return Scaffold( body: Screenshot( controller: screenshotController, child: Text("This text will be captured as an image"), ), ); } }
2. Capturing Screenshots:
screenshotController.capture().then((Uint8List image) { // Capture Done });
3. Capturing Invisible Widgets:
screenshotController.captureFromWidget(Container( padding: const EdgeInsets.all(30.0), decoration: BoxDecoration( border: Border.all(color: Colors.blueAccent, width: 5.0), color: Colors.redAccent, ), child: Text("This is an invisible widget") )).then((capturedImage) { // Handle captured image });
4. Capturing Long Widgets:
int randomItemCount = Random().nextInt(100); var myLongWidget = Column( children: [ for (int i = 0; i < randomItemCount; i++) Text("Tile Index $i"), ], ); screenshotController.captureFromLongWidget( Material( child: myLongWidget, ), delay: Duration(milliseconds: 100), );
5. Saving Images to a Specific Location:
final directory = (await getApplicationDocumentsDirectory()).path; String fileName = DateTime.now().microsecondsSinceEpoch.toString(); screenshotController.captureAndSave( '$directory/$fileName', fileName: fileName, );
6. Sharing Captured Images:
await screenshotController.capture(delay: const Duration(milliseconds: 10)).then((Uint8List image) async { if (image != null) { final directory = await getApplicationDocumentsDirectory(); final imagePath = await File('$/image.png').create(); await imagePath.writeAsBytes(image); await Share.shareFiles([imagePath.path]); } });
7. Note:
For improved image quality, you can adjust the pixelRatio:
screenshotController.capture( pixelRatio: MediaQuery.of(context).devicePixelRatio * 1.5, );
Known Issue:
Platform views, such as Google Maps and the camera, are currently not supported due to technical limitations in Flutter.
Additional Resources: