background_task: Background Processing and Location Updates for Flutter Apps
Published on by Flutter News Hub
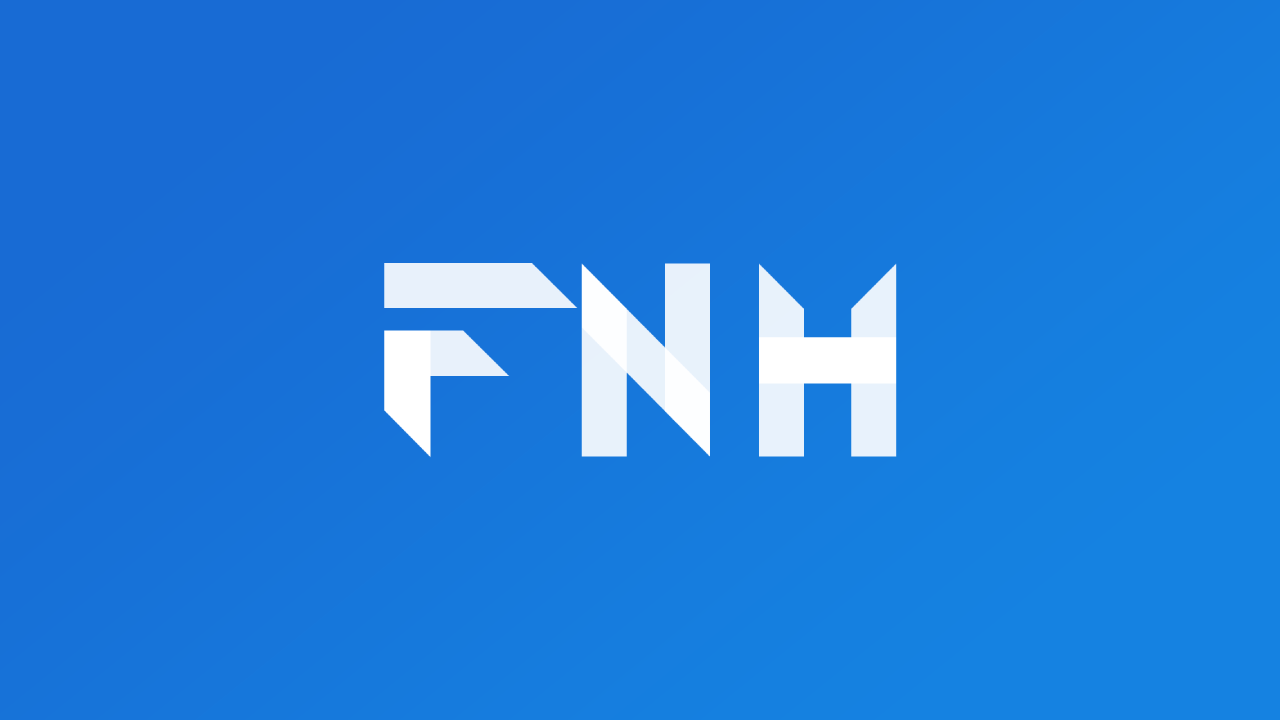
background_task
is a Flutter package that enables developers to continue processing in the background, even when the app transitions to the background. This package is ideal for tasks that require ongoing processing, such as monitoring location updates, sending data to a server, or notifying users of events.
Key Features
- Perform background processing even when the app is in the background
- Receive location updates in the background, even when the app is task-killed
- Set callbacks to handle background tasks, such as processing health data or sending notifications
Usage
To use the background_task
package, follow these steps:
- Install the package:
dependencies:
background_task:
- Import the package:
import 'package:background_task/background_task.dart';
- Start background processing:
await BackgroundTask.instance.start();
- Stop background processing:
await BackgroundTask.instance.stop();
Background Task Execution
To set up a callback handler for background tasks, use the following code:
void main() {
WidgetsFlutterBinding.ensureInitialized();
BackgroundTask.instance.setBackgroundHandler(backgroundHandler); // 👈 Set callback handler
runApp(const MyApp());
}
@pragma('vm:entry-point')
void backgroundHandler(Location data) {
// Implement the process you want to run in the background.
// ex) Check health data.
}
Location Updates in Background
To receive location updates even when the app is task-killed, set the app to Always:
- iOS: Enable Location Always and When In Use Usage Description in Info.plist and add UIBackgroundModes for fetch and location.
- Android: Add permissions for ACCESS_COARSE_LOCATION, ACCESS_FINE_LOCATION, ACCESS_BACKGROUND_LOCATION, FOREGROUND_SERVICE, and POST_NOTIFICATIONS in AndroidManifest.xml.
Enabling Background Task
await BackgroundTask.instance.start(
isEnabledEvenIfKilled: false, // 👈 Disable background task in task-killed status
);
Recommendations
- Use with the
permission_handler
package to handle location permissions. - Register DispatchEngine in AppDelegate for iOS and use ExternalPluginRegistry for Android to use external packages in callback handlers.
Conclusion
The background_task
package provides a robust solution for background processing and location updates in Flutter apps. By leveraging this package, developers can extend the functionality of their apps to perform tasks that require ongoing operation, even when the app is not actively in use.