Assertions in Dart and Flutter Tests: Error Matchers
Published on by Flutter News Hub
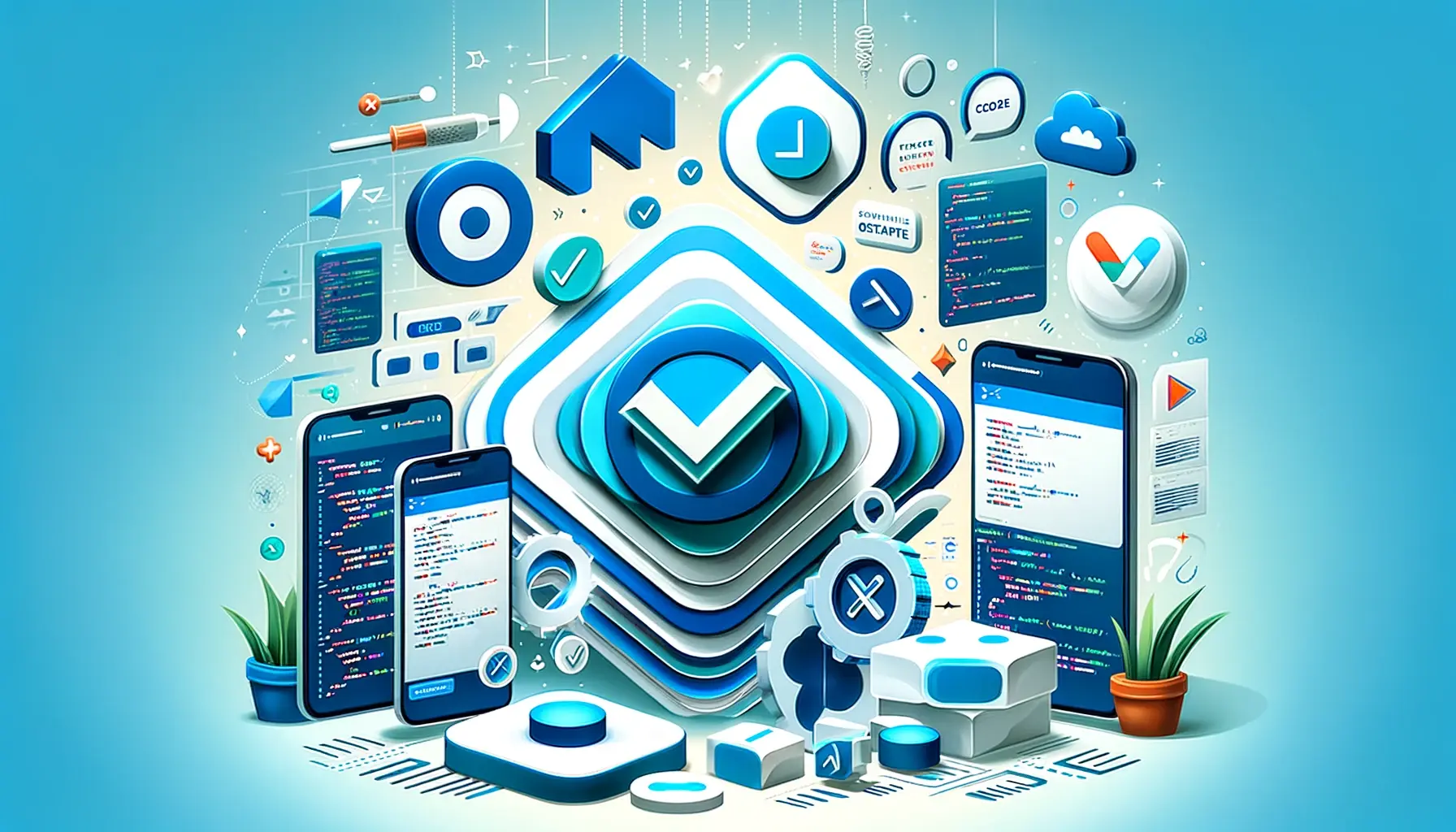
In Flutter testing, it's essential to handle errors gracefully and verify that they occur as expected. Flutter provides a set of error matchers to assert specific types of errors. In this article, we'll explore various error matchers such as isArgumentError, isCastError, isConcurrentModificationError, isCyclicInitializationError, isException, isFormatException, isNoSuchMethodError, isNullThrownError, isRangeError, isStateError, isUnimplementedError, along with explanations and examples for each.
isArgumentError
isArgumentError asserts that an error is an ArgumentError, which occurs when an invalid argument is provided to a function or method.
Example:
test('Throw ArgumentError for invalid argument', () { expect(() => throw ArgumentError(), throwsA(isArgumentError)); });
isConcurrentModificationError
isConcurrentModificationError asserts that an error is a ConcurrentModificationError, which occurs when a collection is modified while an iteration is in progress.
Example:
test('Throw ConcurrentModificationError for concurrent modification', () { var list = [1, 2, 3]; expect(() { for (var item in list) { list.add(4); // Modify the list while iterating } }, throwsA(isConcurrentModificationError)); });
isException
isException asserts that an error is an Exception, a superclass for all exceptions.
Example:
test('Throw Exception for general exception', () { expect(() => throw Exception('Custom exception'), throwsA(isException)); });
isFormatException
isFormatException checks if an error is a FormatException, which occurs when a string cannot be parsed into the expected format.
Example:
test('Throw FormatException for invalid string format', () { expect(() => int.parse('abc'), throwsA(isFormatException)); });
isNoSuchMethodError
isNoSuchMethodError asserts that an error is a NoSuchMethodError, which occurs when a non-existent method or property is accessed.
Example:
test('Throw NoSuchMethodError for accessing non-existent method', () { dynamic car; expect(() => car.nonExistentMethod(), throwsA(isNoSuchMethodError)); });
isRangeError
isRangeError asserts that an error is a RangeError, which occurs when a value is outside the valid range for a method or operation.
Example:
test('Throw RangeError for value out of range', () { expect(() => List<int>.filled(-1, 0), throwsA(isRangeError)); });
isStateError
isStateError checks if an error is a StateError, which occurs when a method is invoked at an illegal or inappropriate time or state.
Example:
test('Throw StateError for illegal state', () { expect(() => [].firstWhere((element) => false), throwsA(isStateError)); });
isUnimplementedError
isUnimplementedError asserts that an error is an UnimplementedError, which occurs when a method or operation is not implemented.
Example:
test('Throw UnimplementedError for unimplemented method', () { expect(() => throw UnimplementedError(), throwsA(isUnimplementedError)); });
Conclusion
These error matchers provide valuable tools for handling and verifying errors in Flutter tests. By leveraging isArgumentError, isCastError, isConcurrentModificationError, isCyclicInitializationError, isException, isFormatException, isNoSuchMethodError, isNullThrownError, isRangeError, isStateError, and isUnimplementedError, developers can ensure their applications behave as expected under various error conditions.