Assertions in Dart and Flutter Tests: Core Matchers
Published on by Flutter News Hub
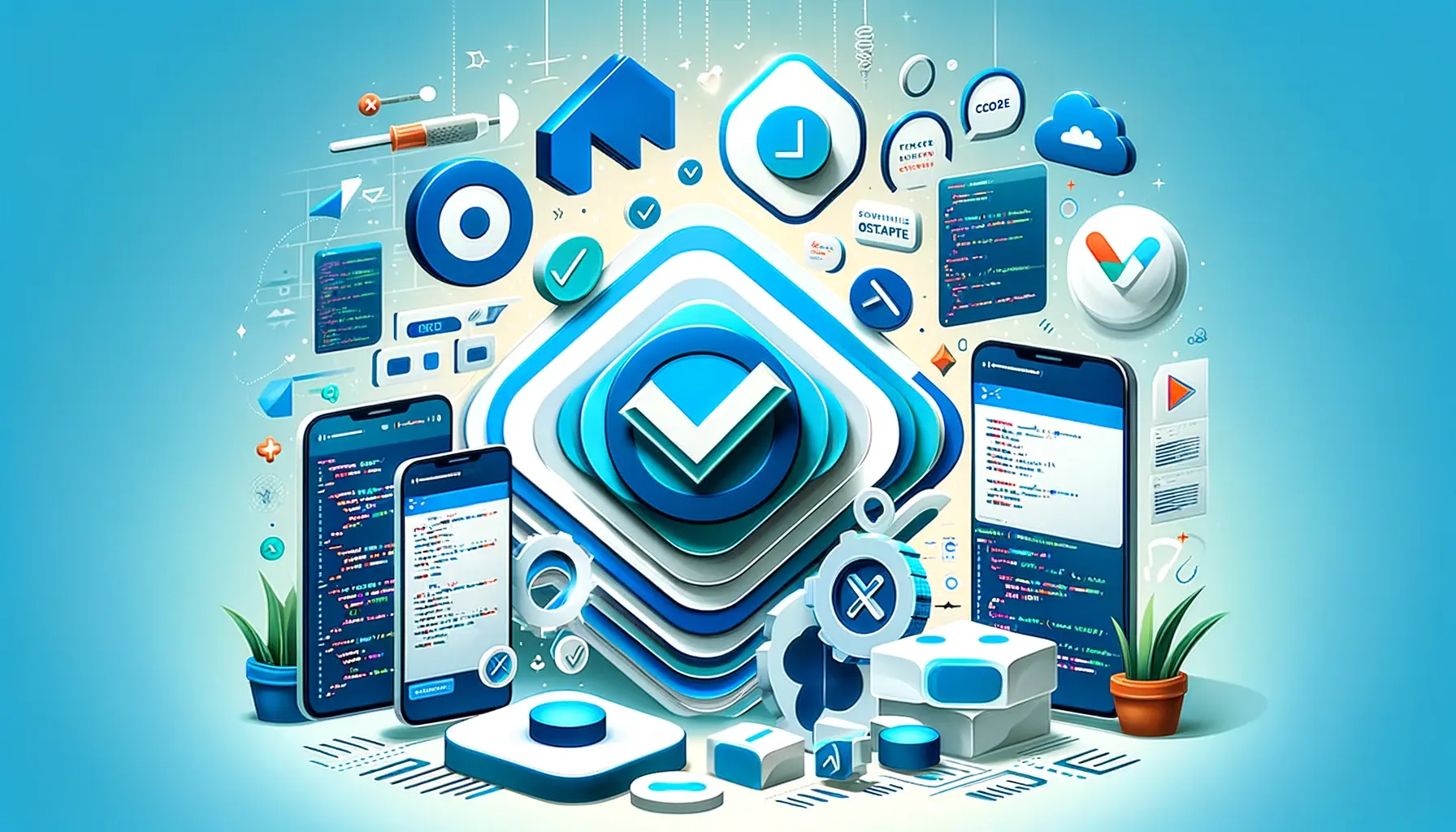
Testing in Flutter is a critical aspect of app development, ensuring your app functions as intended. Understanding the variety of assertions available in Flutter can greatly enhance your testing approach. Below, we explore several key matchers, each accompanied by an explanation and example.
isEmpty
isEmpty is used to verify that a collection or string is empty.
Example:
test('List is empty', () { expect([], isEmpty); });
isNotEmpty
Opposite to isEmpty, isNotEmpty checks if a collection or string has elements.
Example:
test('List is not empty', () { expect(['Flutter'], isNotEmpty); });
isNull
isNull is used to assert that a value is null.
Example:
test('Value is null', () { var value; expect(value, isNull); });
isNotNull
isNotNull asserts that a value is not null.
Example:
test('Value is not null', () { var value = 'Flutter'; expect(value, isNotNull); });
isTrue
isTrue checks if a Boolean value is true.
Example:
test('Boolean value is true', () { expect(true, isTrue); });
isFalse
isFalse asserts that a Boolean value is false.
Example:
test('Boolean value is false', () { expect(false, isFalse); });
isNaN
isNaN checks if a value is Not-a-Number (NaN).
Example:
test('Value is NaN', () { expect(0 / 0, isNaN); });
isNotNaN
isNotNaN asserts that a value is not NaN.
Example:
test('Value is not NaN', () { expect(5 / 2, isNotNaN); });
same
same checks if two references point to the same object.
Example:
test('Two references are the same', () { var object = Object(); expect(object, same(object)); });
isInstanceOf
isInstanceOf<T>() asserts that an object is an instance of a specific type T.
Example:
test('Object is instance of String', () { expect('Flutter', isInstanceOf<String>()); });
isMap
isMap checks if an object is a Map.
Example:
test('Object is a Map', () { expect({'key': 'value'}, isMap); });
isList
isList asserts that an object is a List.
Example:
test('Object is a List', () { expect([1, 2, 3], isList); });
hasLength
hasLength checks if a collection has a specific number of elements.
Example:
test('List has specific length', () { expect(['Flutter', 'Dart'], hasLength(2)); });
isIn
isIn checks if an element is in a collection.
Example:
test('Element is in the list', () { expect('Dart', isIn(['Flutter', 'Dart'])); });
predicate
predicate is used to apply a custom condition.
Example:
test('Custom predicate condition', () { expect(10, predicate<int>((int value) => value > 5)); });
contains
contains checks if a collection or string contains a specified element or substring.
Example:
test('String contains a substring', () { expect('Flutter', contains('utt')); });
returnsNormally
returnsNormally asserts that a function call completes without throwing an exception.
Example:
test('Function returns normally', () { void testFunction() {} expect(testFunction, returnsNormally); });
anything
anything is an all-encompassing matcher that is true for any value except null.
Example:
test('Anything matcher', () { expect('Flutter', anything); });
Conclusion
Mastering these matchers in Flutter testing is key to writing robust and comprehensive tests. Each matcher serves a specific purpose, allowing you to precisely assert various conditions in your app's functionality.