Animated, Responsive, and Reorderable Lists in Flutter Simplified
Published on by Flutter News Hub
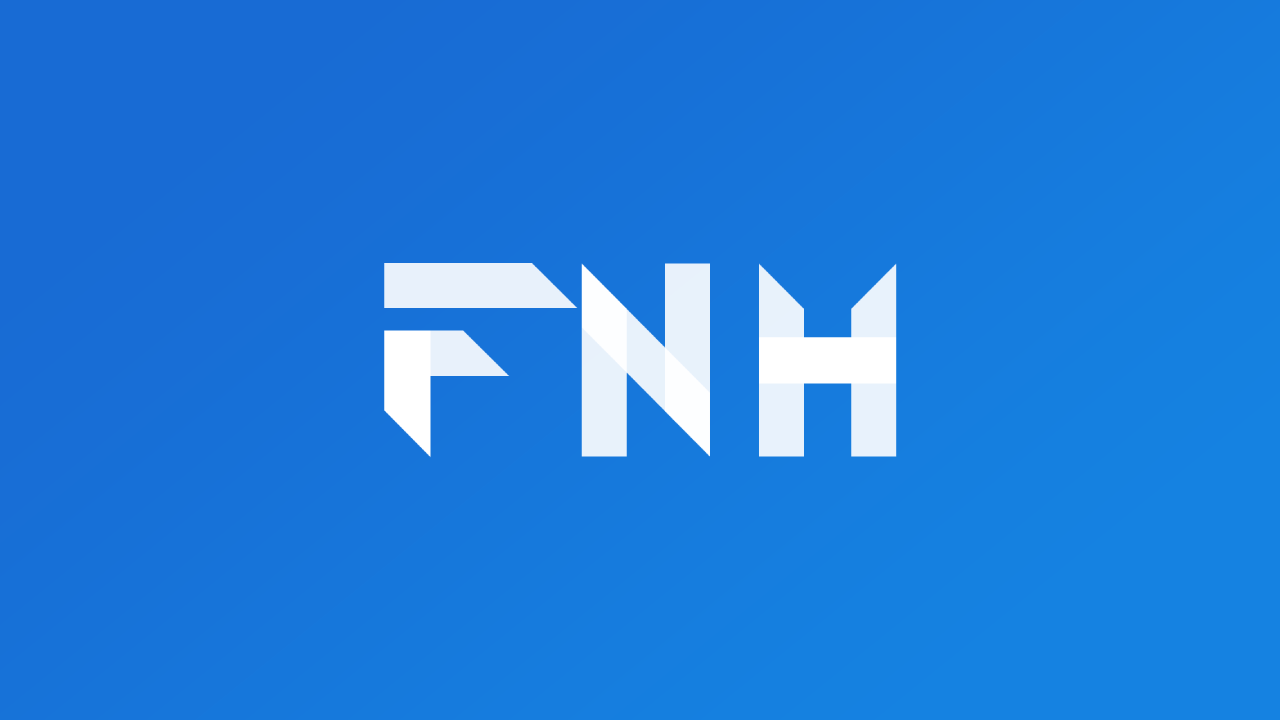
Flutter's Animated Reorderable List is a must-have tool for any developer looking to create sleek, user-friendly lists and grids in their apps. This library combines the power of animation and drag-and-drop functionality, making it a breeze to implement interactive and visually appealing user interfaces.
Getting Started with Animated Reorderable List
To use this library, first add the dependency to your pubspec.yaml
file:
dependencies:
animated_reorderable_list:
Next, import the animated_reorderable_list
package into your Dart file:
import 'package:animated_reorderable_list/animated_reorderable_list.dart';
Animated Reorderable GridView
The AnimatedReorderableGridView
provides drag-and-drop functionality to your grids. Here's an example:
AnimatedReorderableGridView(
items: list,
scrollDirection: Axis.vertical,
itemBuilder: (context, index) {
return ItemCard(
key: Key(list[index].name),
index: list[index].index,
);
},
sliverGridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 4,
),
enterTransition: [
FadeIn(),
ScaleIn(),
],
exitTransition: [
SlideIn(),
],
insertDuration: const Duration(milliseconds: 300),
removeDuration: const Duration(milliseconds: 300),
onReorder: (int oldIndex, int newIndex) {
setState(() {
final User user = list.removeAt(oldIndex);
list.insert(newIndex, user);
});
},
)
Animated Reorderable ListView
Similar to the grid view, the AnimatedReorderableListView
adds drag-and-drop functionality to your lists:
AnimatedReorderableListView(
items: list,
itemBuilder: (context, index) {
return ItemTile(
key: Key(list[index].name),
index: list[index].index,
);
},
enterTransition: [
FlipInX(),
ScaleIn(),
],
exitTransition: [
SlideInLeft(),
],
insertDuration: const Duration(milliseconds: 300),
removeDuration: const Duration(milliseconds: 300),
onReorder: (int oldIndex, int newIndex) {
setState(() {
final User user = list.removeAt(oldIndex);
list.insert(newIndex, user);
});
},
)
Animated ListView and GridView
If you only need animation without drag-and-drop, consider AnimatedListView
and AnimatedGridView
:
AnimatedListView(
items: list,
itemBuilder: (context, index) {
return ItemTile(
key: Key(list[index].name),
index: list[index].index,
);
},
enterTransition: [
FadeIn(),
ScaleIn(),
],
exitTransition: [
SlideIn(),
],
insertDuration: const Duration(milliseconds: 300),
removeDuration: const Duration(milliseconds: 300),
)
AnimatedGridView(
items: list,
scrollDirection: Axis.vertical,
itemBuilder: (context, index) {
return ItemCard(
key: Key(list[index].name),
index: list[index].index,
);
},
sliverGridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 4,
),
enterTransition: [
FadeIn(),
ScaleIn(),
],
exitTransition: [
SlideIn(),
],
insertDuration: const Duration(milliseconds: 300),
removeDuration: const Duration(milliseconds: 300),
)
Customizing Animations
You can tailor the animations to your liking. Here's how to provide your own insertTransition
and removeTransition
:
enterTransition: [
FadeIn(
duration: const Duration(milliseconds: 300),
delay: const Duration(milliseconds: 100),
),
ScaleIn(
duration: const Duration(milliseconds: 500),
curve: Curves.bounceInOut,
),
],
exitTransition: [
SlideIn(
duration: const Duration(milliseconds: 300),
delay: const Duration(milliseconds: 100),
),
ScaleOut(
duration: const Duration(milliseconds: 500),
curve: Curves.bounceInOut,
),
],
Custom Animation Builders
For complete control, use insertItemBuilder
and removeItemBuilder
to create custom animations:
insertItemBuilder: (Widget child, Animation animation) {
return ScaleTransition(
scale: animation,
child: child,
);
},
removeItemBuilder: (Widget child, Animation animation) {
return ScaleTransition(
scale: animation,
child: child,
);
}
Conclusion
With its ease of use, intuitive API, and impressive performance, Animated Reorderable List is the perfect choice for developers who want to enhance their Flutter apps with interactive, animated lists and grids. Whether it's for a chat interface, a photo gallery, or a shopping list, this library provides the tools and flexibility to create stunning and functional user experiences.