Animate Your Flutter Apps with Flutter Animate: A Comprehensive Guide
Published on by Flutter News Hub
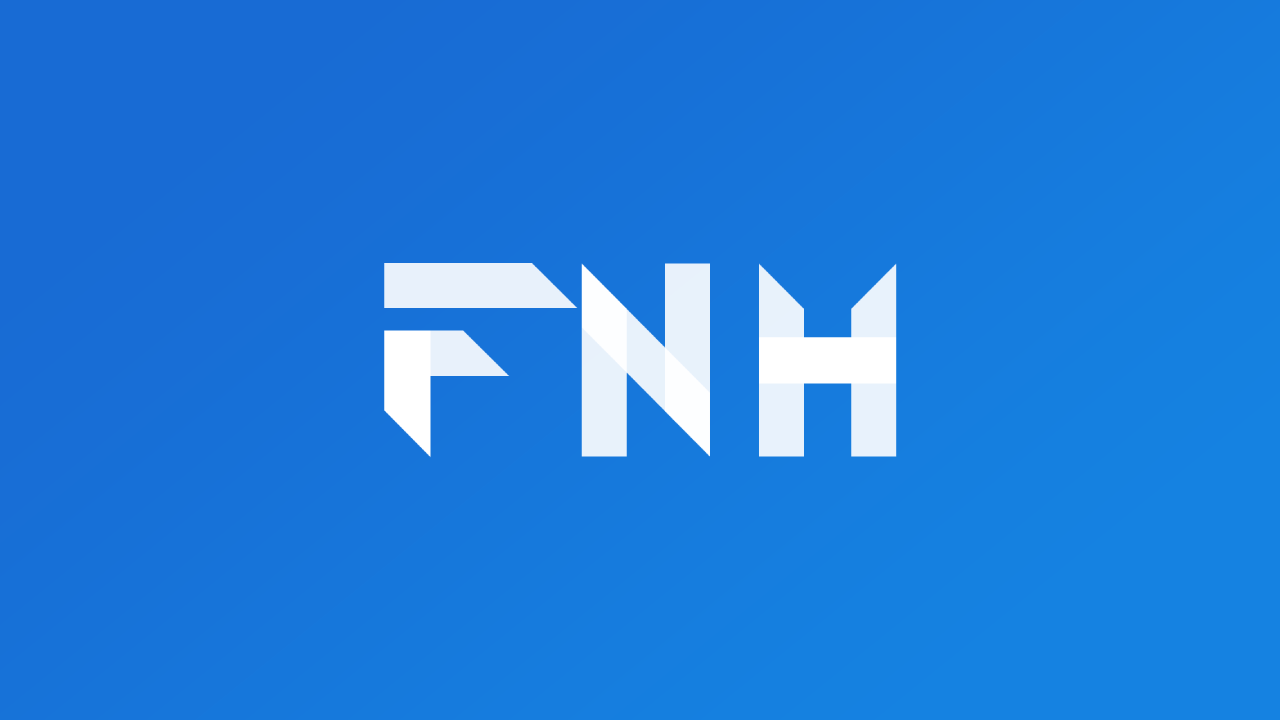
Flutter Animate is a powerful library that simplifies adding stunning animations to your Flutter applications. Here's a comprehensive guide to unlocking its potential.
Basic Syntax
To apply effects to your widget, wrap it in Animate
and specify a list of Effect
instances:
Animate(
effects: [
FadeEffect(),
ScaleEffect(),
],
child: Text("Hello World!"),
);
A handy shorthand syntax is also available:
Text("Hello World!").animate().fade().scale();
Duration, Delay, and Curve
Each effect can have optional delay
, duration
, and curve
parameters:
Text("Hello").animate()
.fade(duration: 500.ms)
.scale(delay: 500.ms); // runs after fade
Effects run concurrently, but delays can create sequential effects.
Sequencing with ThenEffect
ThenEffect
establishes a new baseline time and resets delays for subsequent effects:
Text("Hello").animate()
.fadeIn(duration: 600.ms)
.then(delay: 200.ms) // baseline=800ms
.slide();
Custom Effects and Builders
-
CustomEffect
: Build custom effects using abuilder
function. -
ToggleEffect
: Toggle a boolean value at a specific time. -
SwapEffect
: Swap the target widget at a specified time.
Example:
Text("Before").animate()
.swap(
duration: 900.ms,
builder: (_, __) => Text("After"),
);
Shader Effects
ShaderEffect
enables the application of animated GLSL fragment shaders to widgets:
myWidget.animate()
.shader(duration: 2.seconds, shader: myShader)
.fadeIn(duration: 300.ms);
Events and Callbacks
Animate
offers callbacks for onInit
, onPlay
, and onComplete
.
CallbackEffect
:
Text("Hello").animate()
.fadeIn(duration: 600.ms)
.callback(duration: 300.ms, callback: (_) => print('halfway'));
ListenEffect
:
Text("Hello").animate()
.fadeIn(curve: Curves.easeOutExpo)
.listen(callback: (value) => print('current opacity: $value'));
Adapters and Controllers
-
Adapters: Synchronize animations to external sources (e.g.,
ScrollAdapter
). -
Controllers: Provide your own external
AnimationController
.
Example:
MyButton().animate(target: _over ? 1 : 0)
.fade(end: 0.8)
.scaleXY(end: 1.1);
Testing Animations
Enable automatic animation restart on hot reload:
Animate.restartOnHotReload = true;
Conclusion
Flutter Animate empowers you to seamlessly integrate sophisticated animations into your Flutter projects. With its intuitive API and wide range of effects and features, it simplifies the process of adding visual flair and improving user engagement.