ad_gridview: A Comprehensive Flutter Widget for Seamlessly Integrating Native Ads and Custom Widgets into GridViews
Published on by Flutter News Hub
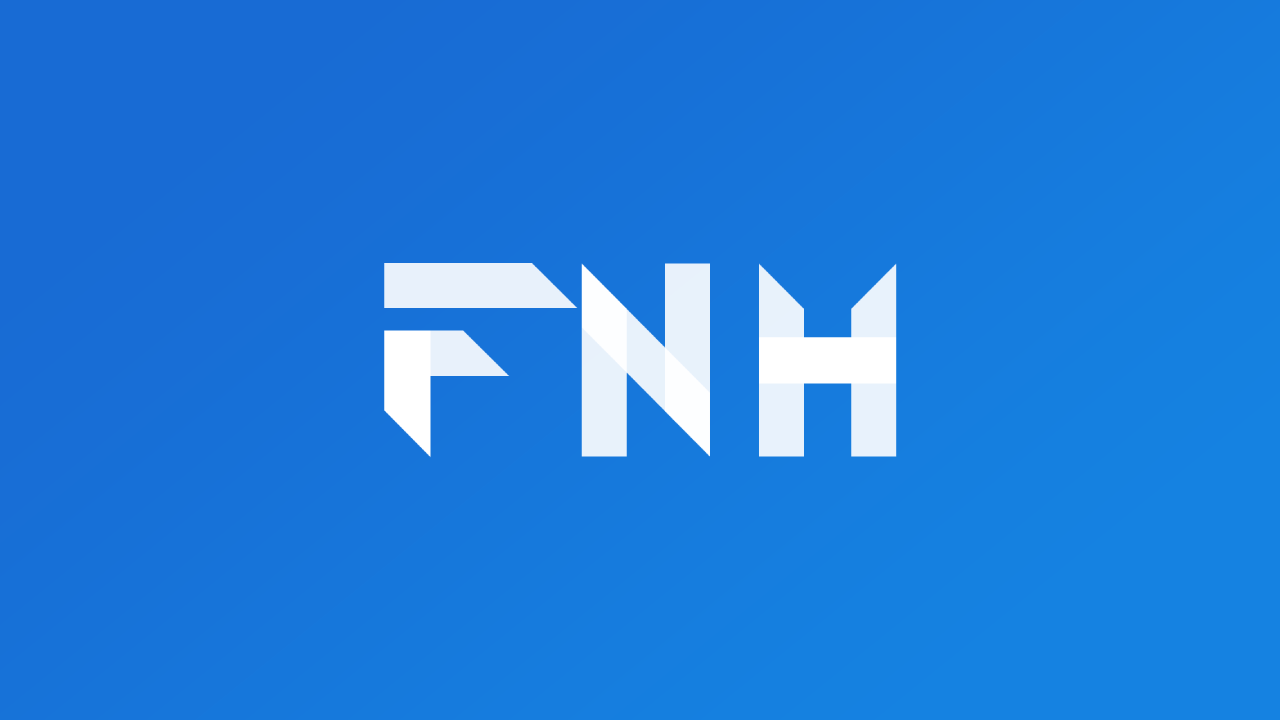
The ad_gridview package provides a convenient and flexible widget for displaying Native Advertisements or any desired Widget within a Flutter GridView. It simplifies the integration process, enabling you to enhance your app's user experience by strategically placing ads or custom content at specific intervals.
Getting Started
To incorporate ad_gridview into your project, add it as a dependency in your pubspec.yaml file:
dependencies:
ad_gridview:
Then, import the package into your library:
import 'package:ad_gridview/ad_gridview.dart';
Usage
The AdGridView widget requires the following parameters:
- crossAxisCount: The number of columns in the GridView.
- itemCount: The total number of items to be displayed, including ads and custom Widgets.
- adIndex: The index at which the ad should appear.
- adWidget: The Widget to be displayed as an ad.
- itemWidget: The Widget to be displayed as a regular item.
An Example
Consider the following example that incorporates a Native Ad into a GridView containing a list of products:
import 'package:flutter/material.dart';
import 'package:ad_gridview/ad_gridview.dart';
// A sample Native Ad widget
class NativeAdWidget extends StatelessWidget {
const NativeAdWidget({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container(
color: Colors.blue,
padding: const EdgeInsets.all(10),
child: const Text('This is a Native Ad'),
);
}
}
// The main GridView using the AdGridView widget
class ProductsGridView extends StatelessWidget {
const ProductsGridView({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return AdGridView(
crossAxisCount: 2, // Two columns in the GridView
itemCount: 100, // 100 items, including ads and product cards
adIndex: 50, // Display an ad at index 50 (after 50 products)
adWidget: const NativeAdWidget(), // The Native Ad widget
itemWidget: const ProductCard(), // A custom ProductCard Widget
);
}
}
In this example, the products will be displayed in a GridView with two columns, and a Native Advertisement will be inserted after the 50th product.
Benefits
- Seamless Integration: Easily integrate Native Ads or any Widget into your GridView layout.
- Flexibility: Choose the index at which you want to place the ad or custom Widget.
- Convenience: Add multiple instances of Widgets to your GridView with minimal effort.
Additional Features
The ad_gridview package offers additional features to enhance its functionality:
- Custom Ad Cell Builder: Define your own custom logic to build the ad cell.
- Item Spacing: Specify the spacing between items in the GridView.
- Error Handling: Handle errors that may occur during the ad fetching process.
Conclusion
The ad_gridview package provides an effective solution for integrating Native Advertisements or any desired Widget into a Flutter GridView. Its simplicity and flexibility make it an ideal choice for developers seeking to enhance their app's user experience and revenue generation.