Accessing the Windows Registry with Ease: A Dart Package Guide
Published on by Flutter News Hub
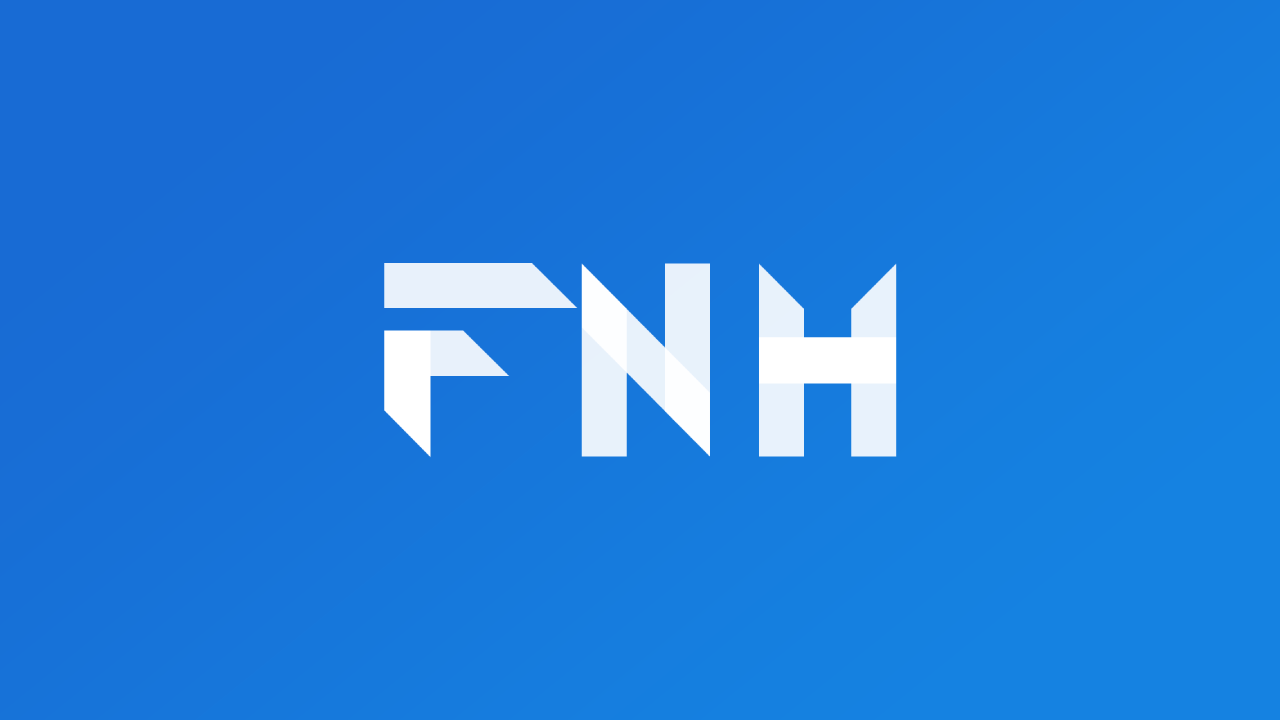
The Windows Registry is a hierarchical database that stores system configurations, application settings, and user preferences in Microsoft Windows operating systems. Traditionally, accessing the registry requires knowledge of the Win32 API and expertise in Foreign Function Interface (FFI). However, with the win32_registry
Dart package, interacting with the registry becomes a breeze.
Getting Started with win32_registry
To start using the win32_registry
package, add it to your pubspec.yaml
file:
dependencies:
win32_registry: ^latest
Once installed, you can import the package into your Dart code:
import 'package:win32_registry/win32_registry.dart';
Opening a Registry Key
To access a specific key in the registry, use the Registry.openPath
method:
final key = Registry.openPath(RegistryHive.localMachine, path: r'Software\Microsoft\Windows NT\CurrentVersion');
The RegistryHive
enum specifies the root of the registry tree:
-
RegistryHive.currentUser
: HKEY_CURRENT_USER -
RegistryHive.localMachine
: HKEY_LOCAL_MACHINE -
RegistryHive.classesRoot
: HKEY_CLASSES_ROOT -
RegistryHive.users
: HKEY_USERS -
RegistryHive.performanceData
: HKEY_PERFORMANCE_DATA
Reading and Writing Registry Values
Once you have a key opened, you can read and write values using the getValue
and setValue
methods:
Reading a string value:
final buildNumber = key.getValueAsString('CurrentBuild');
Writing a string value:
key.setValueAsString('CustomValue', 'Hello, world!');
The setValue
method also supports other value types such as:
-
setValueAsBool
-
setValueAsInt64
-
setValueAsInt32
-
setValueAsDouble
-
setValueAsDateTime
-
setValueAsBinary
Creating and Deleting Registry Keys
You can create new registry keys using the createKey
method:
final newKey = key.createKey('MyNewKey');
To delete a key, use the deleteKey
method:
key.deleteKey('MyNewKey');
Enumerating Registry Keys and Values
To iterate over the keys and values in a registry key, use the getSubkeys
and getValues
methods:
for (final subkey in key.getSubkeys()) {
print('Subkey: ${subkey.path}');
}
for (final value in key.getValues()) {
print('Value: ${value.name} = ${value.value}');
}
Closing Registry Keys
Always remember to close registry keys when you are finished using them to release system resources:
key.close();
Example: Retrieving Windows Build Number
The following example demonstrates retrieving the Windows build number from the registry:
import 'package:win32_registry/win32_registry.dart';
void main() {
const keyPath = r'Software\Microsoft\Windows NT\CurrentVersion';
final key = Registry.openPath(RegistryHive.localMachine, path: keyPath);
final buildNumber = key.getValueAsString('CurrentBuild');
if (buildNumber != null) {
print('Windows build number: $buildNumber');
}
key.close();
}
Key Features
- Cross-platform support for Windows and macOS
- Supports all standard registry data types
- High-level wrapper that simplifies registry access
- Avoids the need for FFI or direct Win32 API knowledge
- Supports both async and synchronous operations
- Fully documented with examples
Conclusion
The win32_registry
package provides a convenient and efficient way to interact with the Windows Registry from Dart applications. With its high-level API and support for all common registry operations, it empowers developers to easily retrieve, modify, and manage registry settings.